使用idea 基于java,用JFrame创建页面,实现简单的,且商品信息能链接数据库的,具有简单购物功能的页面的代码展示
时间: 2024-02-28 17:55:56 浏览: 138
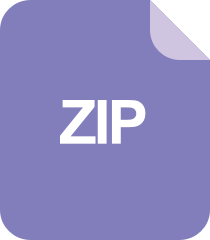
基于Java swing+JFrame+mysql实现的药房销售系统(含源码+数据库+系统程序详细设计文档)
以下是一个简单的 Java Swing 应用程序,它使用 JFrame 创建了一个简单的购物页面,并将商品信息存储在 MySQL 数据库中:
```java
import javax.swing.*;
import java.awt.event.*;
import java.sql.*;
public class ShoppingPage extends JFrame implements ActionListener {
// 商品信息的表格和按钮
private JTable table;
private JButton addButton, removeButton, checkoutButton;
// 数据库连接和语句
private Connection conn;
private Statement stmt;
public ShoppingPage() {
// 创建窗口和布局
super("Shopping Page");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
getContentPane().setLayout(new BoxLayout(getContentPane(), BoxLayout.Y_AXIS));
// 创建表格模型和表格,并添加到窗口中
DefaultTableModel model = new DefaultTableModel(new String[]{"Name", "Price"}, 0);
table = new JTable(model);
getContentPane().add(new JScrollPane(table));
// 创建按钮,并添加到窗口中
addButton = new JButton("Add");
addButton.addActionListener(this);
getContentPane().add(addButton);
removeButton = new JButton("Remove");
removeButton.addActionListener(this);
getContentPane().add(removeButton);
checkoutButton = new JButton("Checkout");
checkoutButton.addActionListener(this);
getContentPane().add(checkoutButton);
// 连接到数据库,并准备语句
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/shopping", "root", "");
stmt = conn.createStatement();
} catch (Exception e) {
e.printStackTrace();
System.exit(1);
}
// 加载商品信息,并显示到表格中
try {
ResultSet rs = stmt.executeQuery("SELECT * FROM products");
while (rs.next()) {
model.addRow(new Object[]{rs.getString("name"), rs.getDouble("price")});
}
} catch (Exception e) {
e.printStackTrace();
System.exit(1);
}
// 显示窗口
pack();
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
// 处理按钮事件
if (e.getSource() == addButton) {
String name = JOptionPane.showInputDialog("Enter product name:");
String price = JOptionPane.showInputDialog("Enter product price:");
try {
stmt.executeUpdate("INSERT INTO products (name, price) VALUES ('" + name + "', " + price + ")");
DefaultTableModel model = (DefaultTableModel) table.getModel();
model.addRow(new Object[]{name, Double.parseDouble(price)});
} catch (Exception ex) {
ex.printStackTrace();
JOptionPane.showMessageDialog(null, "Error adding product.");
}
} else if (e.getSource() == removeButton) {
int row = table.getSelectedRow();
if (row != -1) {
String name = (String) table.getValueAt(row, 0);
try {
stmt.executeUpdate("DELETE FROM products WHERE name = '" + name + "'");
DefaultTableModel model = (DefaultTableModel) table.getModel();
model.removeRow(row);
} catch (Exception ex) {
ex.printStackTrace();
JOptionPane.showMessageDialog(null, "Error removing product.");
}
}
} else if (e.getSource() == checkoutButton) {
double total = 0;
int count = table.getRowCount();
for (int i = 0; i < count; i++) {
double price = (Double) table.getValueAt(i, 1);
total += price;
}
JOptionPane.showMessageDialog(null, "Total price: $" + total);
}
}
public static void main(String[] args) {
new ShoppingPage();
}
}
```
请注意,此代码仅用于演示目的,实际应用程序需要更多的错误检查和安全性措施,以确保数据库和用户数据的安全性。
阅读全文
相关推荐
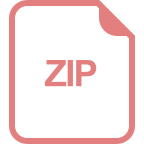
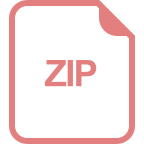















