1.设有学生信息如下:学号(长整型)、姓名(字符串型)、年龄(整型)、课程1成绩(实型)、课程2成绩(实型)、课程3成绩(实型)、课程4成绩(实型)、课程5成绩(实型)、课程6成绩(实型)、课程6成绩(实型)、总分(实型)、平均分(实型)。试编写程序,输入3个学生的上述信息,计算每个学生的总分、平均分,然后输出每个学生学号、姓名、总分、平均分。
时间: 2023-07-04 21:18:39 浏览: 117
好的,这是一个比较典型的数据结构和算法问题,我可以帮你写出这样的一个程序。首先,我们需要定义一个结构体来存储学生的信息:
```
struct student {
long long student_id;
char name[50];
int age;
float score1;
float score2;
float score3;
float score4;
float score5;
float score6;
float total_score;
float avg_score;
};
```
然后,我们可以设计一个函数来输入每个学生的信息:
```
void input_student_info(struct student *s) {
printf("请输入学生的学号:");
scanf("%lld", &(s->student_id));
printf("请输入学生的姓名:");
scanf("%s", s->name);
printf("请输入学生的年龄:");
scanf("%d", &(s->age));
printf("请输入学生的第1门课程成绩:");
scanf("%f", &(s->score1));
printf("请输入学生的第2门课程成绩:");
scanf("%f", &(s->score2));
printf("请输入学生的第3门课程成绩:");
scanf("%f", &(s->score3));
printf("请输入学生的第4门课程成绩:");
scanf("%f", &(s->score4));
printf("请输入学生的第5门课程成绩:");
scanf("%f", &(s->score5));
printf("请输入学生的第6门课程成绩:");
scanf("%f", &(s->score6));
}
```
接着,我们可以设计一个函数来计算每个学生的总分和平均分:
```
void calculate_score(struct student *s) {
s->total_score = s->score1 + s->score2 + s->score3 + s->score4 + s->score5 + s->score6;
s->avg_score = s->total_score / 6.0;
}
```
最后,我们可以设计一个函数来输出每个学生的信息:
```
void output_student_info(struct student *s) {
printf("学生的学号:%lld\n", s->student_id);
printf("学生的姓名:%s\n", s->name);
printf("学生的总分:%f\n", s->total_score);
printf("学生的平均分:%f\n\n", s->avg_score);
}
```
有了以上这些函数,我们就可以编写主函数来输入3个学生的信息,计算总分和平均分,然后输出每个学生的信息:
```
int main() {
struct student s1, s2, s3;
printf("请输入第1个学生的信息:\n");
input_student_info(&s1);
calculate_score(&s1);
printf("请输入第2个学生的信息:\n");
input_student_info(&s2);
calculate_score(&s2);
printf("请输入第3个学生的信息:\n");
input_student_info(&s3);
calculate_score(&s3);
printf("以下是每个学生的信息:\n");
output_student_info(&s1);
output_student_info(&s2);
output_student_info(&s3);
return 0;
}
```
这样,我们就完成了这个程序的编写。你可以自行测试一下,看看是否符合要求。
阅读全文
相关推荐
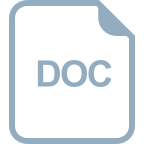
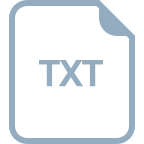
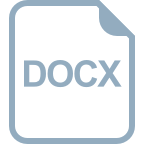













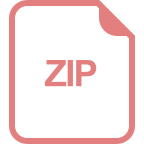
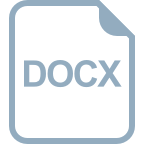
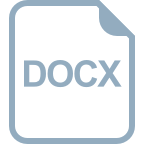