maskrcnn训练自己数据集
时间: 2023-07-06 17:17:44 浏览: 345
Mask R-CNN 是一种基于 Faster R-CNN 的目标检测算法,可以检测出物体的位置,还可以精确地分割出物体的 mask。下面是一个 Mask R-CNN 训练自己数据集的基本步骤:
1. 准备数据集
首先,您需要准备自己的数据集,包括图片和标注。图片可以是任何大小,但最好是统一大小,以便于训练。标注可以使用标准的 COCO 标注格式,包括标注文件和类别文件。
2. 定义类别
您需要定义自己数据集的类别,例如人、车、动物等等。您可以创建一个名为 `CustomConfig` 的类,继承自 `Config` 类,并在其中设置 `NUM_CLASSES` 参数为您的类别数量加 1(因为需要加入一个背景类别):
```python
class CustomConfig(Config):
# Give the configuration a recognizable name
NAME = "custom_dataset"
# Train on 1 GPU and 1 image per GPU
GPU_COUNT = 1
IMAGES_PER_GPU = 1
# Number of classes (including background)
NUM_CLASSES = 1 + 4 # Background + 4 classes
```
3. 定义数据集加载器
您需要定义一个数据集加载器,将准备好的数据集导入到模型中。您可以创建一个名为 `CustomDataset` 的类,继承自 `utils.Dataset` 类,并在其中实现 `load_dataset()`、`load_image()`、`load_mask()`、`image_reference()` 等方法,具体实现可以参考 Mask R-CNN 官方代码。
```python
class CustomDataset(utils.Dataset):
def load_dataset(self, dataset_dir, subset):
self.add_class("custom_dataset", 1, "class1")
self.add_class("custom_dataset", 2, "class2")
self.add_class("custom_dataset", 3, "class3")
self.add_class("custom_dataset", 4, "class4")
# Load annotations
annotations = json.load(open(os.path.join(dataset_dir, "annotations.json")))
annotations = annotations["annotations"]
# Add images and annotations to dataset
for a in annotations:
image_id = a["image_id"]
image_path = os.path.join(dataset_dir, "images", str(image_id) + ".jpg")
if not os.path.exists(image_path):
continue
if a["iscrowd"]:
continue
if a["category_id"] not in [1, 2, 3, 4]:
continue
self.add_image(
"custom_dataset",
image_id=image_id,
path=image_path,
width=a["width"],
height=a["height"],
annotations=a["bbox"]
)
def load_mask(self, image_id):
# Load annotations for image
annotations = self.image_info[image_id]["annotations"]
# Create one mask per instance
masks = np.zeros([self.image_info[image_id]["height"], self.image_info[image_id]["width"], len(annotations)], dtype=np.uint8)
# Load masks
for i, a in enumerate(annotations):
x1, y1, w, h = a
x2 = x1 + w
y2 = y1 + h
masks[y1:y2, x1:x2, i] = 1
# Return masks and class IDs
return masks, np.ones([len(annotations)], dtype=np.int32)
def image_reference(self, image_id):
info = self.image_info[image_id]
return info["path"]
```
4. 训练模型
在训练之前,您需要将预训练 COCO 权重加载到模型中:
```python
model.load_weights(COCO_MODEL_PATH, by_name=True, exclude=["mrcnn_class_logits", "mrcnn_bbox_fc", "mrcnn_bbox", "mrcnn_mask"])
```
然后,您可以使用 `train()` 方法训练模型。在训练之前,您需要创建一个名为 `CustomConfig` 的配置对象,并设置好超参数和文件路径:
```python
config = CustomConfig()
config.display()
model = modellib.MaskRCNN(mode="training", config=config, model_dir=MODEL_DIR)
# Train the head branches
model.train(dataset_train, dataset_val,
learning_rate=config.LEARNING_RATE,
epochs=30,
layers='heads')
```
5. 测试模型
在测试模型之前,您需要将模型切换到 inference 模式:
```python
model = modellib.MaskRCNN(mode="inference", config=config, model_dir=MODEL_DIR)
```
然后,您可以使用 `detect()` 方法对图片进行检测和分割:
```python
results = model.detect([image], verbose=1)
r = results[0]
visualize.display_instances(image, r['rois'], r['masks'], r['class_ids'], class_names, r['scores'])
```
以上就是使用 Mask R-CNN 训练自己数据集的基本步骤。具体实现可以参考 Mask R-CNN 官方代码。
阅读全文
相关推荐
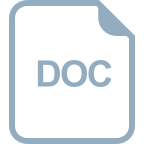
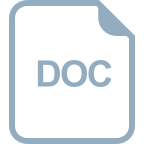
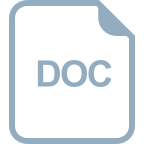

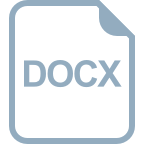
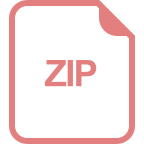







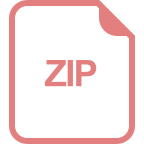




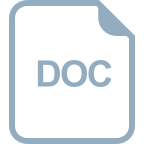