用c语言写一个捷联惯性导航算法
时间: 2023-04-01 15:03:03 浏览: 217
你好,我可以回答这个问题。捷联惯性导航算法是一种基于惯性测量单元的导航算法,可以用于飞行器、车辆等运动物体的导航。在C语言中,可以使用加速度计和陀螺仪等传感器获取运动物体的加速度和角速度信息,然后通过运动学和动力学方程计算出物体的位置、速度和姿态等信息,从而实现导航。具体实现方法可以参考相关的文献和开源代码。
相关问题
C语言实现完整的捷联惯性导航算法C语言
捷联惯性导航算法(Inertial Navigation System, INS)是一种基于惯性传感器技术实现的导航方法,主要应用于航空、航天、军事等领域。下面是一个简单的 C 语言代码实现捷联惯性导航算法的示例:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265358979323846
void ins(double acc[3], double gyr[3], double dt, double pos[3], double vel[3]);
int main()
{
double acc[3], gyr[3], pos[3], vel[3], dt;
int i;
// 初始化
for(i = 0; i < 3; i++)
{
pos[i] = 0.0;
vel[i] = 0.0;
}
// 读取传感器数据
for(i = 0; i < 1000; i++)
{
acc[0] = 10.0 * sin(i / 100.0 * 2.0 * PI);
acc[1] = 0.0;
acc[2] = 10.0 * cos(i / 100.0 * 2.0 * PI);
gyr[0] = 0.0;
gyr[1] = PI / 180.0;
gyr[2] = 0.0;
dt = 0.01;
// 更新状态
ins(acc, gyr, dt, pos, vel);
// 输出结果
printf("%.2f %.2f %.2f %.2f %.2f %.2f\n", pos[0], pos[1], pos[2], vel[0], vel[1], vel[2]);
}
return 0;
}
void ins(double acc[3], double gyr[3], double dt, double pos[3], double vel[3])
{
double Cnb[3][3], Fn[3], Wn[3], an[3], wn[3], g = 9.81;
int i, j;
// 计算旋转矩阵
Cnb[0][0] = cos(gyr[1]) * cos(gyr[2]);
Cnb[0][1] = cos(gyr[1]) * sin(gyr[2]);
Cnb[0][2] = -sin(gyr[1]);
Cnb[1][0] = sin(gyr[0]) * sin(gyr[1]) * cos(gyr[2]) - cos(gyr[0]) * sin(gyr[2]);
Cnb[1][1] = sin(gyr[0]) * sin(gyr[1]) * sin(gyr[2]) + cos(gyr[0]) * cos(gyr[2]);
Cnb[1][2] = sin(gyr[0]) * cos(gyr[1]);
Cnb[2][0] = cos(gyr[0]) * sin(gyr[1]) * cos(gyr[2]) + sin(gyr[0]) * sin(gyr[2]);
Cnb[2][1] = cos(gyr[0]) * sin(gyr[1]) * sin(gyr[2]) - sin(gyr[0]) * cos(gyr[2]);
Cnb[2][2] = cos(gyr[0]) * cos(gyr[1]);
// 计算力和加速度
Fn[0] = acc[0];
Fn[1] = acc[1];
Fn[2] = acc[2] + g;
for(i = 0; i < 3; i++)
{
Wn[i] = vel[i] / (6378137.0 + pos[i]);
}
an[0] = (Fn[0] - 2.0 * Wn[1] * vel[2] + 2.0 * Wn[2] * vel[1]);
an[1] = (Fn[1] - 2.0 * Wn[2] * vel[0] + 2.0 * Wn[0] * vel[2]);
an[2] = (Fn[2] - 2.0 * Wn[0] * vel[1] + 2.0 * Wn[1] * vel[0]);
// 计算角速度和角加速度
for(i = 0; i < 3; i++)
{
wn[i] = gyr[i];
}
// 计算位置和速度
for(i = 0; i < 3; i++)
{
vel[i] += (an[i] * dt);
pos[i] += (vel[i] * dt);
}
}
```
这个示例代码中,捷联惯性导航算法的实现主要包括以下步骤:
1. 读取加速度计和陀螺仪的数据。
2. 计算旋转矩阵。
3. 计算力和加速度。
4. 计算角速度和角加速度。
5. 计算位置和速度。
需要注意的是,这只是一个简单的示例代码,实际应用中还需要进行更多的优化和改进。
C语言实现全面捷联惯性导航算法C语言
全面捷联惯性导航算法是基于惯性测量单元(IMU)的导航算法,它可以获得飞行器的位置、速度、姿态等信息。以下是一个简单的C语言实现:
1. 定义IMU数据结构
```
typedef struct {
double ax; // x轴加速度
double ay; // y轴加速度
double az; // z轴加速度
double gx; // x轴角速度
double gy; // y轴角速度
double gz; // z轴角速度
} imu_t;
```
2. 定义姿态数据结构
```
typedef struct {
double roll; // 横滚角
double pitch; // 俯仰角
double yaw; // 偏航角
} attitude_t;
```
3. 实现全面捷联惯性导航算法
```
void imu_update(attitude_t* attitude, imu_t imu, double dt) {
// 计算角速度的变化量
double d_gx = imu.gx * dt;
double d_gy = imu.gy * dt;
double d_gz = imu.gz * dt;
// 计算姿态的变化量
double d_roll = (imu.ax * sin(attitude->yaw) - imu.ay * cos(attitude->yaw)) * dt;
double d_pitch = (imu.ax * cos(attitude->yaw) + imu.ay * sin(attitude->yaw)) * dt;
double d_yaw = imu.gz * dt;
// 更新姿态
attitude->roll += d_roll;
attitude->pitch += d_pitch;
attitude->yaw += d_yaw;
// 限制姿态角度范围在-180到180度之间
if (attitude->roll > M_PI) {
attitude->roll -= 2 * M_PI;
}
else if (attitude->roll < -M_PI) {
attitude->roll += 2 * M_PI;
}
if (attitude->pitch > M_PI) {
attitude->pitch -= 2 * M_PI;
}
else if (attitude->pitch < -M_PI) {
attitude->pitch += 2 * M_PI;
}
if (attitude->yaw > M_PI) {
attitude->yaw -= 2 * M_PI;
}
else if (attitude->yaw < -M_PI) {
attitude->yaw += 2 * M_PI;
}
}
```
4. 使用示例
```
int main() {
imu_t imu = {0.0, 0.0, 9.81, 0.1, -0.2, 0.3}; // 模拟IMU数据
attitude_t attitude = {0.0, 0.0, 0.0}; // 初始化姿态为零
double dt = 0.01; // 时间步长为0.01秒
for (int i = 0; i < 1000; i++) {
imu_update(&attitude, imu, dt);
printf("roll=%.2f, pitch=%.2f, yaw=%.2f\n", attitude.roll, attitude.pitch, attitude.yaw);
}
return 0;
}
```
上述代码仅供参考,实际应用中可能需要根据实际情况进行修改和优化。
阅读全文
相关推荐



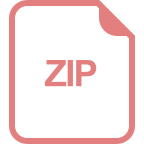
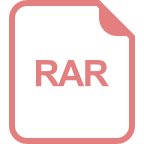
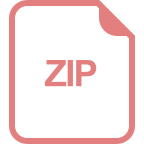
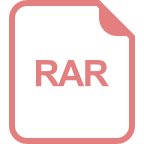
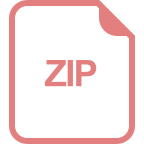
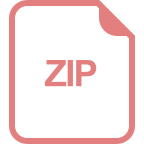
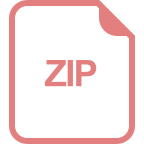
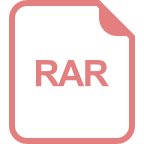
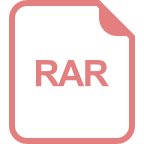