用C++设计一个学生类Student(属性有学号、姓名、3门课成绩<课程编号为1-3>)和教师类Teacher(属性有职工号和姓名),实现以下要求: (1)输出学生成绩; (2)教师可以修改学生成绩。
时间: 2023-06-24 18:06:38 浏览: 78
以下是使用C++设计的学生类Student和教师类Teacher的代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
string name; // 姓名
int id; // 学号
double scores[3]; // 3门课成绩
public:
Student(string n, int i, double s1, double s2, double s3) {
name = n;
id = i;
scores[0] = s1;
scores[1] = s2;
scores[2] = s3;
}
void printScores() {
cout << "学生信息:" << endl;
cout << "姓名:" << name << endl;
cout << "学号:" << id << endl;
cout << "成绩:" << endl;
for (int i = 0; i < 3; i++) {
cout << "课程" << i+1 << "成绩:" << scores[i] << endl;
}
}
void modifyScores(int course_id, double new_score) {
scores[course_id-1] = new_score;
}
};
class Teacher {
private:
string name; // 姓名
int id; // 职工号
public:
Teacher(string n, int i) {
name = n;
id = i;
}
void modifyStudentScores(Student& stu, int course_id, double new_score) {
stu.modifyScores(course_id, new_score);
cout << "教师信息:" << endl;
cout << "姓名:" << name << endl;
cout << "职工号:" << id << endl;
cout << "修改学生成绩成功!" << endl;
}
};
int main() {
Student stu("小明", 1001, 80, 90, 75);
Teacher tea("张老师", 2001);
stu.printScores();
tea.modifyStudentScores(stu, 2, 95); // 修改小明的第2门课成绩为95
stu.printScores();
return 0;
}
```
以上代码定义了一个学生类Student和教师类Teacher,其中学生类有姓名、学号和3门课程成绩属性,教师类有姓名和职工号属性。学生类中实现了输出学生成绩和修改学生成绩的方法,教师类中实现了修改学生成绩的方法。
在主函数中创建了一个学生对象和一个教师对象,先输出学生对象的成绩,然后教师对象调用修改学生成绩的方法修改学生对象的第2门课程成绩为95,最后再输出学生对象的成绩,可以看到成绩已经被修改成功。
相关推荐
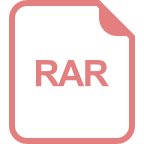
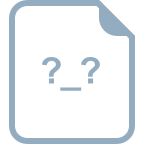
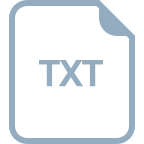














