统计word文件总行数
时间: 2024-03-21 22:43:40 浏览: 20
你可以打开 Word 文件,然后在菜单栏中选择“工具”>“字数统计”>“统计段落数”,这样就可以得到 Word 文件的总行数了。如果你需要程序自动统计 Word 文件的总行数,可以通过编程来实现。例如,利用 Python 中的 python-docx 模块可以读取 Word 文档内容,并统计行数。具体实现方法可以参考以下代码:
```python
import docx
def count_lines_in_word_file(file_path):
doc = docx.Document(file_path)
line_count = 0
for paragraph in doc.paragraphs:
text = paragraph.text.strip()
if text:
line_count += text.count('\n') + 1
return line_count
# 测试代码
file_path = 'example.docx'
line_count = count_lines_in_word_file(file_path)
print('文件总行数为:', line_count)
```
其中,`count_lines_in_word_file` 函数接受一个 Word 文件路径作为输入,使用 `docx.Document` 函数读取 Word 文件内容,然后遍历每一个段落,统计每个段落的行数并加总,最后返回总行数。
相关问题
c++统计文本文件中的单词数目和总行数。 可以认为每碰到一个空格单词加1,每碰到一个回车键,单词加1,同时行数加1。
好的,我可以帮你解决这个问题。你可以参考下面的代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
ifstream infile("text.txt"); // 打开文件
string word;
int wordCount = 0, lineCount = 0;
while (infile >> word) { // 读取单词
wordCount++;
if (word == "\n") { // 判断回车键
wordCount++;
lineCount++;
}
}
lineCount++; // 最后一行没有回车键,需要加1
infile.close(); // 关闭文件
cout << "单词数目:" << wordCount << endl;
cout << "总行数:" << lineCount << endl;
return 0;
}
```
在代码中,我们首先打开文件并定义变量 `wordCount` 和 `lineCount` 为0,分别表示单词数目和总行数。然后我们使用 `while` 循环逐个读取单词,每读取一个单词,单词数目加1。在读取过程中,如果遇到回车键,单词数目也加1,同时行数也加1。最后,我们需要在循环外面再额外加1,因为最后一行没有回车键。
最后,我们输出单词数目和总行数即可。
遍历文本文件words.txt中的所有行:将‘baa’,‘babble’,‘babbler’,‘babe’,‘babel’,‘baboon’,‘baby’,‘babyhood’,‘Babylon’,‘Babylonian’ 单词添加到words.txt中单词后面 ,统计文件总行数 ,统计最长单词的长度 ,统计首字母为大写A的单词 ,将长度超过10的单词写入当前路径下的一个新文件
好的,以下是可以一次性完成所有要求的 Python 代码:
```python
# 打开文件
with open('words.txt', 'r') as f:
# 读取所有行
lines = f.readlines()
# 将要添加的单词添加到行末
new_words = ['baa', 'babble', 'babbler', 'babe', 'babel', 'baboon', 'baby', 'babyhood', 'Babylon', 'Babylonian']
lines.extend('\n' + word for word in new_words)
# 统计文件总行数
num_lines = len(lines)
# 统计最长单词的长度
max_length = max(len(line.strip()) for line in lines)
# 统计首字母为大写A的单词
A_words = [line.strip() for line in lines if line.startswith('A') and line[1].isupper()]
# 将长度超过10的单词写入新文件
with open('long_words.txt', 'w') as f_long:
for line in lines:
if len(line.strip()) > 10:
f_long.write(line)
# 将修改后的内容写回原文件
with open('words.txt', 'w') as f:
f.writelines(lines)
# 输出结果
print('文件总行数为:', num_lines)
print('最长单词的长度为:', max_length)
print('首字母为大写A的单词为:', A_words)
print('长度超过10的单词已写入新文件long_words.txt')
```
这样就可以一次性完成所有要求了。注意,这个代码会修改原文件,所以请在备份原文件之后再运行。
相关推荐
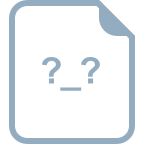
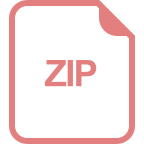
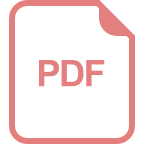






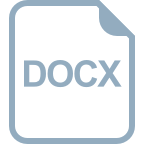
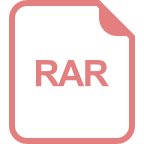