C语言:输入n个字符(不超过81,回车(换行)结束),分别统计出字母,空格,数字字符,和其他字符的个数
时间: 2024-05-05 11:20:14 浏览: 57
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char ch;
int letter_count = 0, space_count = 0, digit_count = 0, other_count = 0;
while ((ch = getchar()) != '\n') {
if (isalpha(ch)) {
letter_count++;
} else if (isspace(ch)) {
space_count++;
} else if (isdigit(ch)) {
digit_count++;
} else {
other_count++;
}
}
printf("字母个数:%d\n", letter_count);
printf("空格个数:%d\n", space_count);
printf("数字字符个数:%d\n", digit_count);
printf("其他字符个数:%d\n", other_count);
return 0;
}
```
注:使用 `ctype.h` 库中的 `isalpha()`、`isspace()`、`isdigit()` 函数可以判断一个字符是否是字母、空格、数字字符。
相关问题
C语言题目描述 输入一行字符,分别统计出其中英文字母、空格、数字和其他字符的个数。 输入 输入一行字符,以回车符结束 输出 分别输出字母、空格、数字和其它字符的个数
这道题目要求我们输入一行字符,然后统计其中的英文字母、空格、数字和其他字符的个数。下面是C语言的代码实现:
```c
#include <stdio.h>
int main() {
char ch;
int space = 0, number = 0, character = 0, other = 0;
ch = getchar(); // 字符输入
while (ch != '\n') { // '\n'是回车
if (ch == ' ') { // 字符ch为空格
space++;
} else if (ch >= '0' && ch <= '9') { // 字符为数字0~9
number++;
} else if (ch >= 'a' && ch <= 'z' || ch >= 'A' && ch <= 'Z') { // 字符为字母a~z,A~Z
character++;
} else {
other++;
}
ch = getchar();
}
printf("%d %d %d %d", character, space, number, other);
return 0;
}
```
这段代码中,我们使用了getchar()函数来输入字符,然后使用while循环来遍历输入的字符。在循环中,我们使用if语句来判断字符的类型,然后分别统计字母、空格、数字和其他字符的个数。最后,我们使用printf()函数输出结果。
4.统计英文字母、空格或换行、数字字符:输入一个正整数n ,再输入n 个字符,统计其中英文字母、空格或回车、数字字符和其他字符的个数。试编写相应程序。用C语言编写
以下是用C语言编写的程序:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
int n, letter = 0, space = 0, digit = 0, other = 0;
char c;
printf("请输入正整数n:");
scanf("%d", &n);
for (int i = 1; i <= n; i++) {
printf("请输入第%d个字符:", i);
scanf(" %c", &c); // 注意加入空格,避免读入上一次输入中的回车符
if (isalpha(c)) { // 判断是否为英文字母
letter++;
} else if (isspace(c)) { // 判断是否为空格或回车
space++;
} else if (isdigit(c)) { // 判断是否为数字字符
digit++;
} else { // 其他字符
other++;
}
}
printf("输入的字符串中包含英文字母%d个,空格或回车%d个,数字字符%d个,其他字符%d个。\n", letter, space, digit, other);
return 0;
}
```
程序中使用了循环语句for来输入n个字符,并根据字符的属性统计英文字母、空格或回车、数字字符和其他字符的个数。最后输出统计结果。在判断字符属性时,使用了C标准库中的isalpha、isspace和isdigit函数。
阅读全文
相关推荐
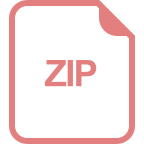













