% 计算单应性矩阵 H = homography2d(original_points, target_points);
时间: 2024-05-16 11:14:00 浏览: 11
在计算单应性矩阵之前,需要先确定原始点和目标点的坐标。然后使用以下代码计算单应性矩阵H:
```
import numpy as np
from numpy.linalg import inv
def homography2d(original_points, target_points):
num_points = original_points.shape[0]
A = np.zeros((2*num_points, 9))
for i in range(num_points):
x, y = original_points[i, 0], original_points[i, 1]
u, v = target_points[i, 0], target_points[i, 1]
A[2*i] = np.array([-x, -y, -1, 0, 0, 0, x*u, y*u, u])
A[2*i+1] = np.array([0, 0, 0, -x, -y, -1, x*v, y*v, v])
U, S, V = np.linalg.svd(A)
H = V[-1].reshape((3, 3))
H = (1/H[2, 2]) * H
return H
```
其中,原始点和目标点分别是大小为N×2的二维数组,N表示点的数量。函数返回的是单应性矩阵H,大小为3×3的矩阵。
相关问题
H = homography2d(original_points, target_points);函数定义函数体MATLAB
function H = homography2d(original_points, target_points)
% HOMOGRAPHY2D Computes the homography between two sets of 2D points
% H = HOMOGRAPHY2D(ORIGINAL_POINTS, TARGET_POINTS) computes the
% homography between two sets of 2D points using the Direct Linear
% Transform (DLT) algorithm.
%
% INPUTS:
% original_points - 2xN matrix of N original 2D points
% target_points - 2xN matrix of N target 2D points
%
% OUTPUTS:
% H - 3x3 homography matrix that maps original points to target points
% Check inputs
assert(size(original_points, 1) == 2, 'original_points should be a 2xN matrix');
assert(size(target_points, 1) == 2, 'target_points should be a 2xN matrix');
assert(size(original_points, 2) == size(target_points, 2), 'original_points and target_points should have the same number of points');
% Normalize points
[original_points_normalized, T_original] = normalize_points(original_points);
[target_points_normalized, T_target] = normalize_points(target_points);
% Construct matrix A
N = size(original_points_normalized, 2);
A = zeros(2*N, 9);
for i=1:N
A(2*i-1, :) = [0, 0, 0, -original_points_normalized(1,i), -original_points_normalized(2,i), -1, target_points_normalized(2,i)*original_points_normalized(1,i), target_points_normalized(2,i)*original_points_normalized(2,i), target_points_normalized(2,i)];
A(2*i, :) = [original_points_normalized(1,i), original_points_normalized(2,i), 1, 0, 0, 0, -target_points_normalized(1,i)*original_points_normalized(1,i), -target_points_normalized(1,i)*original_points_normalized(2,i), -target_points_normalized(1,i)];
end
% Solve Ah = 0 using SVD
[~, ~, V] = svd(A);
h = V(:, end);
% Reshape h to obtain H
H = reshape(h, 3, 3)';
% Denormalize H
H = T_target \ H * T_original;
end
function [points_normalized, T] = normalize_points(points)
% NORMALIZE_POINTS Normalizes 2D points to have zero mean and unit standard deviation
% [POINTS_NORMALIZED, T] = NORMALIZE_POINTS(POINTS) normalizes 2D points
% to have zero mean and unit standard deviation by scaling and translating
% the points according to the formula: x' = (x - mu) / sigma, where x is
% the original point, x' is the normalized point, mu is the mean of all
% points and sigma is the standard deviation of all points. The function
% returns the normalized points and the transformation matrix T that has
% to be applied to the points to obtain their normalized version.
% Compute mean and standard deviation of points
mu = mean(points, 2);
sigma = std(points, 0, 2);
% Compute transformation matrix
T = [1/sigma(1) 0 -mu(1)/sigma(1); 0 1/sigma(2) -mu(2)/sigma(2); 0 0 1];
% Apply transformation matrix to points
points_normalized = T * [points; ones(1, size(points, 2))];
% Discard last row of ones
points_normalized = points_normalized(1:2, :);
end
H = homography2d(original_points, target_points);在MATLAB中使用报错
这个错误可能是由于以下原因引起的:
1. homography2d 函数不存在。请确认您是否正确地输入了函数名称,并且该函数确实存在于您的 MATLAB 环境中。
2. 输入参数错误。请确保您正确地输入了原始点和目标点参数,它们应该是两个 n × 2 的矩阵。
3. 数据类型错误。请确保输入的矩阵的数据类型是双精度浮点型。
4. 数据维度错误。请确保原始点和目标点矩阵具有相同的行数和列数。
如果您无法解决问题,请提供更多的代码和错误信息,这样我们才能更好地帮助您解决问题。
相关推荐
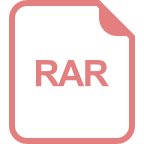












