DSP和nRF24L01的代码
时间: 2024-05-03 17:17:06 浏览: 119
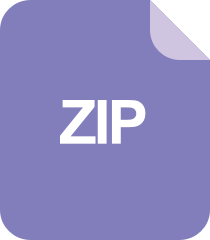
nRF24L01代码
以下是DSP和nRF24L01的代码示例:
DSP代码示例:
```c
#include <stdio.h>
void main()
{
int a = 5, b = 10, c;
c = a + b;
printf("Sum of a and b is %d ", c);
}
```
nRF24L01代码示例:
```c
#include <SPI.h>
#include <nRF24L01.h>
#include <RF24.h>
RF24 radio(9, 10); // CE, CSN
const uint64_t pipe = 0xE8E8F0F0E1LL;
void setup() {
Serial.begin(9600);
radio.begin();
radio.setChannel(115);
radio.openReadingPipe(1, pipe);
radio.startListening();
}
void loop() {
if (radio.available()) {
char text[32] = "";
radio.read(&text, sizeof(text));
Serial.println(text);
}
}
```
请注意,这些代码只是示例,具体的代码实现可能需要更多的代码来完成所需的任务。此外,nRF24L01的代码示例是使用RF24库编写的,因此您需要将该库添加到您的Arduino IDE中以使用它。
阅读全文
相关推荐
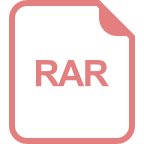
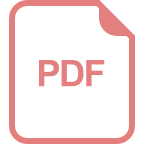
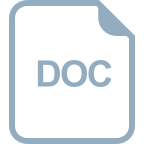

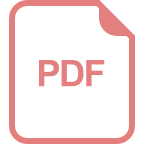
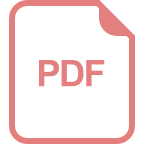
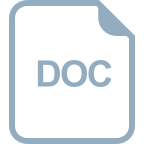
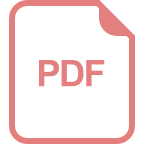
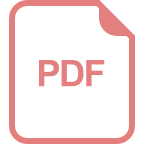
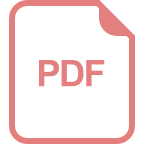
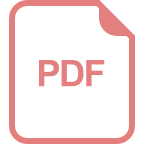
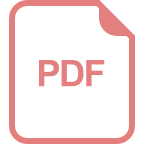
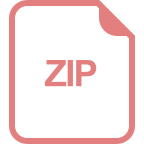
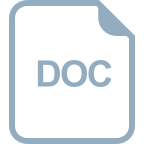
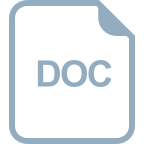
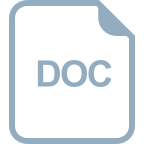