vue实现2行的方格,从鼠标按下触发的方格开始,拖动选中多个方格,到鼠标弹起触发的方格结束,只可选择一段且连续的方格。选中的放格颜色为红色,未选中为黑色
时间: 2023-04-04 22:00:46 浏览: 85
可以通过以下代码实现:
在 HTML 中:
```
<div id="app">
<div v-for="(row, rowIndex) in rows" :key="rowIndex" class="row">
<div v-for="(col, colIndex) in row" :key="colIndex" :class="{'col': true, 'selected': isSelected(rowIndex, colIndex)}" @mousedown="startSelection(rowIndex, colIndex)" @mouseover="select(rowIndex, colIndex)" @mouseup="endSelection">
{{ col }}
</div>
</div>
</div>
```
在 JavaScript 中:
```
new Vue({
el: '#app',
data: {
rows: [
['A', 'B', 'C', 'D', 'E'],
['F', 'G', 'H', 'I', 'J'],
['K', 'L', 'M', 'N', 'O'],
['P', 'Q', 'R', 'S', 'T'],
['U', 'V', 'W', 'X', 'Y']
],
startRow: null,
startCol: null,
endRow: null,
endCol: null
},
methods: {
startSelection(row, col) {
this.startRow = row;
this.startCol = col;
this.endRow = row;
this.endCol = col;
},
select(row, col) {
if (this.startRow !== null && this.startCol !== null) {
this.endRow = row;
this.endCol = col;
}
},
endSelection() {
this.startRow = null;
this.startCol = null;
this.endRow = null;
this.endCol = null;
},
isSelected(row, col) {
if (this.startRow === null || this.startCol === null || this.endRow === null || this.endCol === null) {
return false;
}
const minRow = Math.min(this.startRow, this.endRow);
const maxRow = Math.max(this.startRow, this.endRow);
const minCol = Math.min(this.startCol, this.endCol);
const maxCol = Math.max(this.startCol, this.endCol);
return row >= minRow && row <= maxRow && col >= minCol && col <= maxCol;
}
}
});
```
在 CSS 中:
```
.row {
display: flex;
}
.col {
width: 50px;
height: 50px;
border: 1px solid black;
text-align: center;
line-height: 50px;
}
.selected {
background-color: red;
}
```
阅读全文
相关推荐
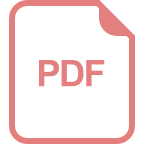
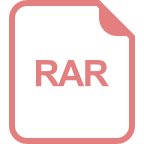
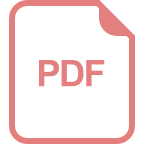


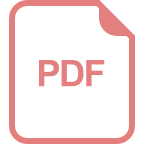
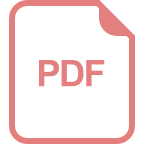
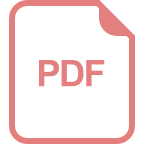
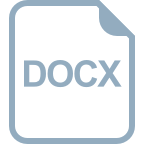
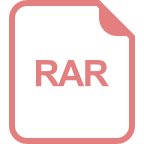
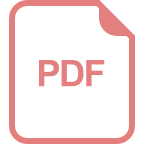
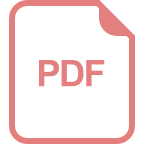
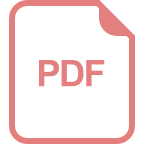
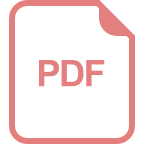
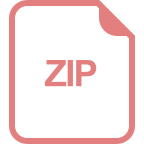
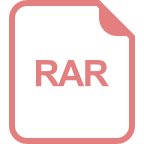