写一个共享内存用于进程间通信
时间: 2023-12-13 16:02:54 浏览: 75
共享内存是一种进程间通信方式,允许多个进程访问同一块内存区域。下面是一个使用 POSIX 共享内存的示例代码:
```c
#include <sys/mman.h>
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#define SHM_NAME "/myshm"
#define SHM_SIZE 4096
int main() {
int fd = shm_open(SHM_NAME, O_CREAT | O_RDWR, 0666); // 创建共享内存文件描述符
if (fd < 0) {
perror("shm_open");
exit(1);
}
if (ftruncate(fd, SHM_SIZE) < 0) { // 调整文件大小
perror("ftruncate");
exit(1);
}
void *addr = mmap(NULL, SHM_SIZE, PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0); // 映射到进程内存空间
if (addr == MAP_FAILED) {
perror("mmap");
exit(1);
}
close(fd); // 关闭文件描述符
pid_t pid = fork();
if (pid < 0) {
perror("fork");
exit(1);
} else if (pid == 0) { // 子进程
printf("Child process is writing to shared memory...\n");
char *str = "Hello, shared memory!";
strncpy(addr, str, strlen(str)); // 写入数据
exit(0);
} else { // 父进程
wait(NULL);
printf("Parent process read from shared memory: %s\n", (char*)addr); // 读取数据
munmap(addr, SHM_SIZE); // 解除映射
shm_unlink(SHM_NAME); // 删除共享内存
}
return 0;
}
```
在此示例中,首先使用 `shm_open` 创建共享内存文件描述符,并使用 `ftruncate` 调整文件大小。然后使用 `mmap` 将共享内存映射到进程内存空间中。子进程通过指针 `addr` 访问共享内存并写入数据。父进程等待子进程完成后,读取共享内存中的数据。最后,使用 `munmap` 解除映射并使用 `shm_unlink` 删除共享内存。
阅读全文
相关推荐
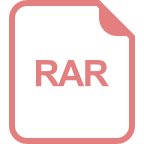











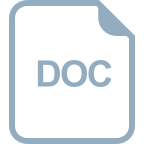

