用c++编写的学生信息管理系统
时间: 2023-09-27 11:05:51 浏览: 49
以下是一个简单的学生信息管理系统的C++代码示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
// 定义学生结构体
struct Student {
string name;
int age;
string gender;
string major;
};
int main() {
// 打开文件
ofstream outfile("students.txt", ios::app);
// 写入学生信息
Student s;
cout << "请输入学生姓名:";
cin >> s.name;
cout << "请输入学生年龄:";
cin >> s.age;
cout << "请输入学生性别:";
cin >> s.gender;
cout << "请输入学生专业:";
cin >> s.major;
outfile << s.name << " " << s.age << " " << s.gender << " " << s.major << endl;
// 关闭文件
outfile.close();
return 0;
}
```
以上示例中,我们定义了一个学生结构体,包含了学生的姓名、年龄、性别和专业。然后我们通过文件流将学生信息保存到名为“students.txt”的文件中。
如果需要查询学生信息,可以通过读取文件来实现。以下是一个简单的读取学生信息的C++代码示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
// 定义学生结构体
struct Student {
string name;
int age;
string gender;
string major;
};
int main() {
// 打开文件
ifstream infile("students.txt");
// 读取学生信息
Student s;
while (infile >> s.name >> s.age >> s.gender >> s.major) {
cout << "姓名:" << s.name << endl;
cout << "年龄:" << s.age << endl;
cout << "性别:" << s.gender << endl;
cout << "专业:" << s.major << endl;
}
// 关闭文件
infile.close();
return 0;
}
```
以上示例中,我们通过文件流读取名为“students.txt”的文件,并将学生信息输出到控制台上。
相关推荐







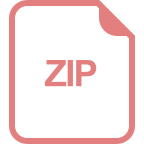
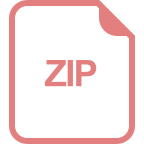
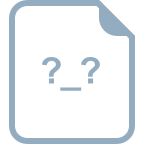
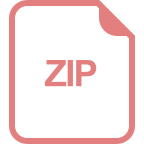