python和sql server连接
时间: 2023-04-27 20:00:27 浏览: 49
Python可以通过pyodbc库来连接SQL Server数据库。需要先安装pyodbc库,然后使用以下代码连接数据库:
```python
import pyodbc
# 连接数据库
conn = pyodbc.connect('DRIVER={SQL Server};SERVER=服务器地址;DATABASE=数据库名称;UID=用户名;PWD=密码')
# 执行SQL语句
cursor = conn.cursor()
cursor.execute('SELECT * FROM 表名')
# 获取查询结果
result = cursor.fetchall()
# 关闭连接
cursor.close()
conn.close()
```
其中,需要将`服务器地址`、`数据库名称`、`用户名`和`密码`替换为实际的值。执行SQL语句可以使用`execute()`方法,获取查询结果可以使用`fetchall()`方法。最后需要关闭连接,释放资源。
相关问题
python怎么和sqlserver连接
Python可以使用pyodbc模块来连接SQL Server数据库。具体步骤如下:
1. 安装pyodbc模块
可以使用pip命令来安装pyodbc模块:
```
pip install pyodbc
```
2. 导入pyodbc模块
在Python代码中导入pyodbc模块:
```
import pyodbc
```
3. 连接SQL Server数据库
使用pyodbc.connect()函数来连接SQL Server数据库,需要指定数据库的连接字符串,例如:
```
conn = pyodbc.connect('DRIVER={SQL Server};SERVER=服务器名称;DATABASE=数据库名称;UID=用户名;PWD=密码')
```
其中,DRIVER指定使用的驱动程序,SQL Server指定连接的数据库类型,SERVER指定服务器名称,DATABASE指定数据库名称,UID和PWD分别指定用户名和密码。
4. 执行SQL语句
连接成功后,可以使用cursor()方法创建游标对象,然后使用execute()方法执行SQL语句,例如:
```
cursor = conn.cursor()
cursor.execute('SELECT * FROM 表名')
```
5. 获取查询结果
可以使用fetchall()方法获取查询结果,例如:
```
result = cursor.fetchall()
```
6. 关闭连接
使用close()方法关闭连接,例如:
```
conn.close()
```
python 连接sql server
### 回答1:
Python可以使用pyodbc模块连接SQL Server数据库。具体步骤如下:
1. 安装pyodbc模块
可以使用pip命令进行安装:
```
pip install pyodbc
```
2. 导入pyodbc模块
```
import pyodbc
```
3. 连接SQL Server数据库
使用pyodbc.connect()方法连接数据库,需要提供数据库的连接信息,例如:
```
server = 'localhost'
database = 'testdb'
username = 'sa'
password = 'password'
cnxn = pyodbc.connect('DRIVER={SQL Server};SERVER='+server+';DATABASE='+database+';UID='+username+';PWD='+ password)
```
4. 执行SQL语句
可以使用cursor对象执行SQL语句,例如:
```
cursor = cnxn.cursor()
cursor.execute("SELECT * FROM table_name")
```
5. 获取查询结果
可以使用fetchall()方法获取查询结果,例如:
```
rows = cursor.fetchall()
for row in rows:
print(row)
```
以上就是使用Python连接SQL Server数据库的基本步骤。
### 回答2:
Python是一种非常流行的编程语言,拥有大量的库和框架,能够处理各种数据,包括SQL Server数据库。下面介绍如何使用Python来连接SQL Server。
第一步,安装PyODBC库。PyODBC是Python与ODBC(开放数据库连接)之间的接口。在安装PyODBC之前,需要确保已安装ODBC驱动程序。可以使用多种方法安装PyODBC,比如pip install pyodbc。
第二步,导入pyodbc库,并创建与SQL Server数据库的连接。具体代码如下:
import pyodbc
conn = pyodbc.connect("DRIVER={ODBC Driver 17 for SQL Server};"
"SERVER=myserver.database.windows.net;"
"DATABASE=mydb;"
"UID=myusername;"
"PWD=mypassword")
在这个例子中,使用ODBC Driver 17 for SQL Server驱动程序与SQL Server建立连接。将相应的服务器名、数据库名、用户名和密码替换为实际值。
第三步,执行SQL查询。可以使用游标将SQL查询发送到服务器,并获得结果。
cursor = conn.cursor()
cursor.execute('SELECT * FROM mytable')
data = cursor.fetchall()
以上代码将从表mytable中选择所有行,并将它们存储在一个变量data中。可以使用其他SQL语句来执行其他操作,如插入、更新或删除数据。
第四步,关闭连接。
cursor.close()
conn.close()
以上是Python连接SQL Server的基本流程。可以使用其他库和框架来扩展这个过程,如使用pandas库来将查询结果转换为数据框。在连接SQL Server时,需要注意一些安全性问题,如使用强密码、限制访问权限等措施。
### 回答3:
Python可以通过两种方式连接SQL Server,一种是使用ODBC驱动程序,另一种是使用pyodbc包。接下来将分别介绍这两种方法的实现步骤。
1. 使用ODBC连接SQL Server
(1)安装ODBC驱动程序
首先需要安装ODBC驱动程序,可选择SQL Server Native 10.0或SQL Server Native 11.0版本,这里以SQL Server Native 11.0版本为例。
(2)创建数据源
打开“ODBC数据源管理员”,在“用户DSN”或“系统DSN”中创建一个新数据源,选择SQL Server Native 11.0作为驱动程序,并配置连接字符串、用户名和密码等信息。
(3)编写Python程序
导入pyodbc包,使用connect()方法连接ODBC数据源,传入数据源名称和用户名密码等参数,然后可以使用cursor()方法执行SQL语句。
示例代码:
```python
import pyodbc
conn = pyodbc.connect('DSN=SQLServer;UID=sa;PWD=123456')
cursor = conn.cursor()
cursor.execute('SELECT * FROM [dbo].[table_name]')
rows = cursor.fetchall()
```
2. 使用pyodbc包连接SQL Server
pyodbc是Python库,提供了与ODBC数据库的连接。可以使用pyodbc包连接SQL Server数据库。
(1)安装pyodbc包
在终端运行以下命令:
```python
pip install pyodbc
```
(2)编写Python程序
导入pyodbc包,使用connect()方法连接SQL Server数据库,传入数据库名称、服务器地址、用户名密码等参数,然后可以使用cursor()方法执行SQL语句。
示例代码:
```python
import pyodbc
conn = pyodbc.connect('Driver={SQL Server};Server=servername;Database=dbname;UID=user;PWD=password')
cursor = conn.cursor()
cursor.execute('SELECT * FROM [dbo].[table_name]')
rows = cursor.fetchall()
```
以上是连接SQL Server的两种方法,通过使用ODBC驱动程序或pyodbc包,可以轻松连接SQL Server数据库,并操作数据。
相关推荐
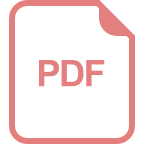
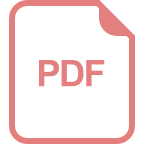












