python编写kmeans算法样例
时间: 2023-07-02 20:07:07 浏览: 38
以下是使用Python编写KMeans算法的样例:
```python
import numpy as np
import matplotlib.pyplot as plt
def kmeans(X, K, max_iters=100):
centroids = X[np.random.choice(len(X), K, replace=False)]
for i in range(max_iters):
clusters = [[] for _ in range(K)]
for x in X:
distances = [np.linalg.norm(x-c) for c in centroids]
cluster_idx = np.argmin(distances)
clusters[cluster_idx].append(x)
prev_centroids = centroids
centroids = [np.mean(cluster, axis=0) for cluster in clusters]
if np.allclose(prev_centroids, centroids):
break
return centroids, clusters
# 生成数据
np.random.seed(0)
X = np.vstack([
np.random.randn(100, 2) + np.array([2, 2]),
np.random.randn(100, 2) + np.array([-2, -2]),
np.random.randn(100, 2) + np.array([-2, 2])
])
# 运行KMeans算法
centroids, clusters = kmeans(X, 3)
# 可视化结果
colors = ['r', 'g', 'b']
for i, cluster in enumerate(clusters):
for x in cluster:
plt.scatter(x[0], x[1], c=colors[i])
for c in centroids:
plt.scatter(c[0], c[1], marker='x', s=200, linewidths=3, color='k')
plt.show()
```
在这个样例中,我们使用Numpy和Matplotlib库来生成数据和可视化结果。在`kmeans()`函数中,我们首先随机初始化K个质心,然后迭代地将每个点分配到最近的质心,并根据分配结果重新计算质心位置。当质心位置不再变化时,算法停止迭代并返回质心和聚类结果。最后,我们使用Matplotlib库将数据点和质心可视化。
相关推荐
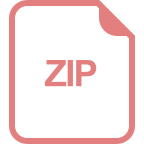














