python爬取网页图片保存
时间: 2023-07-30 19:09:14 浏览: 84
可以使用Python中的requests和BeautifulSoup库来爬取网页图片并保存。
首先,使用requests库发送一个GET请求获取网页内容,然后使用BeautifulSoup库解析网页内容,找到所有需要下载的图片的URL。
接下来,使用requests库发送一个GET请求下载图片,并将其保存到本地。
以下是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com' # 网页链接
response = requests.get(url) # 发送GET请求获取网页内容
soup = BeautifulSoup(response.text, 'html.parser') # 解析网页内容
# 找到所有需要下载的图片的URL
image_urls = []
for img in soup.find_all('img'):
image_urls.append(img.get('src'))
# 下载并保存图片
for url in image_urls:
response = requests.get(url)
with open(url.split('/')[-1], 'wb') as f:
f.write(response.content)
```
在上面的示例代码中,将下载的图片保存在当前工作目录下,并以图片的文件名作为文件名保存。
相关问题
python爬取网页图片并保存到本地
在Python中,可以使用requests库获取网页内容,BeautifulSoup库解析HTML,然后使用PIL库处理图像,最后将图片保存到本地。以下是简单的步骤:
1. 安装必要的库:
```bash
pip install requests beautifulsoup4 pillow
```
2. 使用示例代码爬取图片:
```python
import os
import requests
from bs4 import BeautifulSoup
from io import BytesIO
def download_images(url):
# 发送HTTP请求获取页面内容
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
# 解析HTML
soup = BeautifulSoup(response.text, 'html.parser')
# 找到所有img标签
img_tags = soup.find_all('img')
for img_tag in img_tags:
# 获取图片链接
img_url = img_tag['src']
# 下载图片
try:
img_data = requests.get(img_url).content
# 创建文件名,可以根据需求添加日期、哈希等信息
file_name = os.path.join('images', os.path.basename(img_url))
with open(file_name, 'wb') as f:
f.write(img_data)
print(f"图片 {file_name} 已下载")
except Exception as e:
print(f"无法下载图片 {img_url}: {e}")
# 用你要爬取的网址替换下面的url
download_images("http://example.com") # 替换为实际网站URL
Python爬取网页图片
你可以使用 Python 中的 requests 库和 BeautifulSoup 库来爬取网页图片。以下是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 目标网页 URL
url = 'https://www.example.com'
# 发送 GET 请求并获取响应
response = requests.get(url)
# 解析 HTML 页面
soup = BeautifulSoup(response.text, 'html.parser')
# 找到所有的图片标签
img_tags = soup.find_all('img')
# 遍历图片标签并下载图片
for img_tag in img_tags:
# 获取图片链接
img_url = img_tag['src']
# 发送 GET 请求并获取图片内容
img_response = requests.get(img_url)
# 将图片内容写入本地文件
with open('image.jpg', 'wb') as f:
f.write(img_response.content)
```
这段代码会将目标网页中的所有图片下载下来并保存在本地文件中。你可以根据实际需求对代码进行修改和优化。
阅读全文
相关推荐
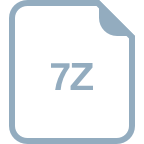










