RCNN代码
时间: 2023-07-02 16:08:37 浏览: 67
RCNN(Region-based Convolutional Neural Networks)是一种基于区域的卷积神经网络,主要用于目标检测任务。以下是RCNN的代码实现(仅供参考):
1. 首先导入必要的库和模块
```python
import numpy as np
import tensorflow as tf
import cv2
import os
import time
from sklearn.externals import joblib
from skimage.feature import hog
from sklearn.svm import LinearSVC
from sklearn.preprocessing import LabelEncoder
from sklearn.model_selection import train_test_split
```
2. 定义函数来提取图像的HOG特征
```python
def extract_features(image_path):
image = cv2.imread(image_path)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
hog_features = hog(gray, orientations=9, pixels_per_cell=(8, 8), cells_per_block=(2, 2), block_norm='L2-Hys')
return hog_features
```
3. 加载训练数据集,并提取HOG特征
```python
train_dir = 'path/to/train/directory'
data = []
labels = []
for folder in os.listdir(train_dir):
label = folder
folder_path = os.path.join(train_dir, folder)
for image_name in os.listdir(folder_path):
image_path = os.path.join(folder_path, image_name)
features = extract_features(image_path)
data.append(features)
labels.append(label)
```
4. 对标签进行编码,并将数据集划分为训练集和测试集
```python
le = LabelEncoder()
labels_encoded = le.fit_transform(labels)
data_train, data_test, labels_train, labels_test = train_test_split(data, labels_encoded, test_size=0.25, random_state=42)
```
5. 使用线性支持向量机(Linear SVM)来训练模型
```python
svm = LinearSVC()
svm.fit(data_train, labels_train)
```
6. 加载测试图像,并用滑动窗口方法在图像上移动,使用训练好的模型来检测目标物体
```python
test_image_path = 'path/to/test/image'
test_image = cv2.imread(test_image_path)
for scale in np.linspace(0.1, 1.0, 10)[::-1]:
resized_image = cv2.resize(test_image, (int(test_image.shape[1] * scale), int(test_image.shape[0] * scale)))
for (x, y, window) in sliding_window(resized_image, step_size=16, window_size=(64, 64)):
if window.shape[0] != 64 or window.shape[1] != 64:
continue
features = extract_features(window)
prediction = svm.predict(features.reshape(1, -1))
if prediction == 1:
x = int(x / scale)
y = int(y / scale)
w = int(64 / scale)
h = int(64 / scale)
cv2.rectangle(test_image, (x, y), (x + w, y + h), (0, 255, 0), 2)
```
以上是RCNN的简单实现,实际上RCNN还有更多的细节和优化。如果需要进行更深入的学习和研究,可以参考相关的论文和代码实现。
相关推荐











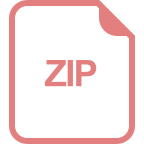