用pygame绘制一个可旋转的立方体
时间: 2023-03-19 10:23:23 浏览: 205
好的,以下是用pygame绘制一个可旋转的立方体的步骤:
1. 导入pygame模块
```python
import pygame
```
2. 初始化pygame
```python
pygame.init()
```
3. 设置窗口大小和标题
```python
size = (640, 480)
screen = pygame.display.set_mode(size)
pygame.display.set_caption("旋转的立方体")
```
4. 定义立方体的顶点坐标和面
```python
vertices = [(100,100,100), (100,-100,100), (-100,-100,100), (-100,100,100), (100,100,-100), (100,-100,-100), (-100,-100,-100), (-100,100,-100)]
faces = [(0,1,2,3), (0,4,5,1), (1,5,6,2), (2,6,7,3), (0,3,7,4), (4,7,6,5)]
```
5. 定义旋转角度和旋转中心
```python
angle = 0
center = (size[0]/2, size[1]/2)
```
6. 定义旋转函数
```python
def rotate_point(point, angle):
x = point[0]
y = point[1]
z = point[2]
cos_a = pygame.math.cos(angle)
sin_a = pygame.math.sin(angle)
new_x = x * cos_a - y * sin_a
new_y = x * sin_a + y * cos_a
new_z = z
return (new_x, new_y, new_z)
```
7. 定义绘制函数
```python
def draw_cube(vertices, faces, angle):
for face in faces:
pointlist = []
for i in face:
point = vertices[i]
point = rotate_point(point, angle)
x = point[0] + center[0]
y = point[1] + center[1]
pointlist.append((x, y))
pygame.draw.polygon(screen, (255,255,255), pointlist)
```
8. 进入游戏循环,不断旋转并绘制立方体
```python
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((0,0,0))
draw_cube(vertices, faces, angle)
angle += 0.01
pygame.display.flip()
```
以上就是用pygame绘制一个可旋转的立方体的完整代码。
阅读全文
相关推荐






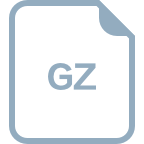





