Tensorflow手写数字识别代码
时间: 2023-11-17 18:05:27 浏览: 90
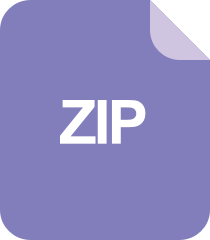
机器学习课程作业-基于tensorflow编写的对手写数字图像识别源码(使用notebook).zip
以下是使用Tensorflow实现手写数字识别的代码:
```python
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
# 加载数据集
mnist = tf.keras.datasets.mnist
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
# 数据预处理
train_images = train_images / 255.0
test_images = test_images / 255.0
# 定义模型
model = tf.keras.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dropout(0.2),
tf.keras.layers.Dense(10)
])
# 编译模型
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
# 训练模型
model.fit(train_images, train_labels, epochs=10)
# 评估模型
test_loss, test_acc = model.evaluate(test_images, test_labels, verbose=2)
print('\nTest accuracy:', test_acc)
# 预测新样本
probability_model = tf.keras.Sequential([model, tf.keras.layers.Softmax()])
predictions = probability_model.predict(test_images)
# 显示预测结果
plt.figure(figsize=(10, 10))
for i in range(25):
plt.subplot(5, 5, i+1)
plt.xticks([])
plt.yticks([])
plt.grid(False)
plt.imshow(test_images[i], cmap=plt.cm.binary)
predicted_label = np.argmax(predictions[i])
true_label = test_labels[i]
if predicted_label == true_label:
color = 'green'
else:
color = 'red'
plt.xlabel("{} ({})".format(predicted_label, true_label), color=color)
plt.show()
```
该代码使用了一个包含两个隐藏层的神经网络模型,其中第一个隐藏层包含128个神经元,并使用ReLU激活函数。为防止过拟合,我们使用了一个Dropout层。模型训练时使用的损失函数是交叉熵,优化器是Adam。在训练完成后,我们对测试集进行评估,并使用Softmax函数对预测结果进行标准化处理。最后,我们显示了一些测试集中的样本以及它们的预测结果。
阅读全文
相关推荐
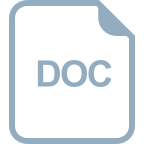
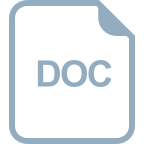
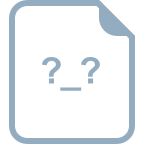
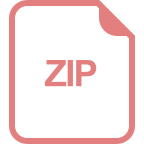
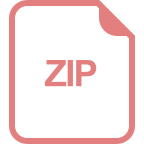
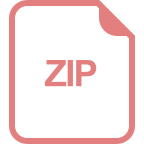
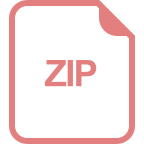
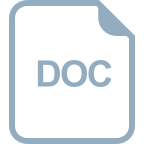
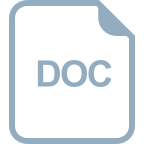
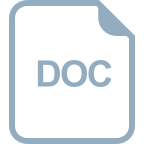
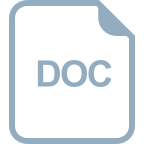



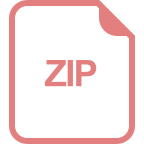

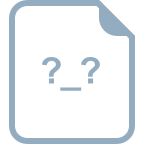