java实现不带头结点的单链表
时间: 2024-04-30 07:17:46 浏览: 7
以下是Java实现不带头结点的单链表的代码:
```java
public class SinglyLinkedList {
private Node head;
private class Node {
private int data;
private Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
public SinglyLinkedList() {
this.head = null;
}
public boolean isEmpty() {
return head == null;
}
public void insertFirst(int data) {
Node newNode = new Node(data);
newNode.next = head;
head = newNode;
}
public void insertLast(int data) {
Node newNode = new Node(data);
if (isEmpty()) {
head = newNode;
return;
}
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
public Node deleteFirst() {
if (isEmpty()) {
return null;
}
Node temp = head;
head = head.next;
return temp;
}
public void displayList() {
Node current = head;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
System.out.println();
}
}
```
这里使用了内部类`Node`来表示链表中的节点,其中`data`表示节点存储的数据,`next`表示指向下一个节点的引用。`head`表示链表的头节点,初始值为`null`。链表的操作包括:判断链表是否为空、在链表头部插入节点、在链表尾部插入节点、删除链表头部节点、打印整个链表。
相关推荐
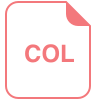













