用C++实现职工工资管理要求代码完整且能运行 基本要求: 定义职工(employee)类,其中至少包括姓名、性别、工号、电话、所在科室和工资。 功能要求: 1、设计菜单实现功能选择: 2、输入功能:输入职工信息,并保存到文件中: 3、查询功能: 1)能够根据工号精确查询职工信息: 2)能够根据姓名、科室查询职工信息 3)分科室进行工资统计,计算各科室的平均工资 4、根据职工的工资排序输出 5、根据工号修改职工信息 6、根据工号删除职工信息
时间: 2023-07-10 09:12:11 浏览: 176
以下是一个简单的职工工资管理系统的C++代码,满足您的基本要求和功能要求:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
using namespace std;
// 声明职工类
class Employee {
public:
string name; // 姓名
string gender; // 性别
int id; // 工号
string phone; // 电话
string department; // 所在科室
double salary; // 工资
// 构造函数
Employee(string _name="", string _gender="", int _id=0, string _phone="", string _department="", double _salary=0.0) {
name = _name;
gender = _gender;
id = _id;
phone = _phone;
department = _department;
salary = _salary;
}
// 输出职工信息
friend ostream& operator<< (ostream& out, const Employee& employee) {
out << employee.name << " " << employee.gender << " " << employee.id << " " << employee.phone << " " << employee.department << " " << employee.salary << endl;
return out;
}
// 输入职工信息
friend istream& operator>> (istream& in, Employee& employee) {
in >> employee.name >> employee.gender >> employee.id >> employee.phone >> employee.department >> employee.salary;
return in;
}
// 按工资从小到大排序
bool operator< (const Employee& other) const {
return salary < other.salary;
}
};
// 声明职工管理类
class EmployeeManager {
private:
vector<Employee> employees; // 职工列表
// 保存职工信息到文件
void saveToFile() {
ofstream out("employees.txt");
for (Employee& employee : employees) {
out << employee;
}
out.close();
}
// 从文件加载职工信息
void loadFromFile() {
ifstream in("employees.txt");
Employee employee;
while (in >> employee) {
employees.push_back(employee);
}
in.close();
}
public:
// 构造函数
EmployeeManager() {
loadFromFile();
}
// 菜单
void showMenu() {
cout << "请选择功能:" << endl;
cout << "1. 输入职工信息" << endl;
cout << "2. 精确查询职工信息" << endl;
cout << "3. 模糊查询职工信息" << endl;
cout << "4. 统计各科室平均工资" << endl;
cout << "5. 按工资排序输出" << endl;
cout << "6. 修改职工信息" << endl;
cout << "7. 删除职工信息" << endl;
cout << "8. 退出" << endl;
}
// 输入职工信息
void addEmployee() {
string name, gender, phone, department;
int id;
double salary;
cout << "请输入职工姓名:";
cin >> name;
cout << "请输入职工性别:";
cin >> gender;
cout << "请输入职工工号:";
cin >> id;
cout << "请输入职工电话:";
cin >> phone;
cout << "请输入职工所在科室:";
cin >> department;
cout << "请输入职工工资:";
cin >> salary;
Employee employee(name, gender, id, phone, department, salary);
employees.push_back(employee);
cout << "职工信息已保存" << endl;
saveToFile();
}
// 精确查询职工信息
void exactQueryEmployee() {
int id;
cout << "请输入要查询的职工工号:";
cin >> id;
for (Employee& employee : employees) {
if (employee.id == id) {
cout << employee;
return;
}
}
cout << "未找到该职工信息" << endl;
}
// 模糊查询职工信息
void fuzzyQueryEmployee() {
string name, department;
cout << "请输入要查询的职工姓名:";
cin >> name;
cout << "请输入要查询的职工所在科室:";
cin >> department;
bool found = false;
for (Employee& employee : employees) {
if (employee.name == name && employee.department == department) {
cout << employee;
found = true;
}
}
if (!found) {
cout << "未找到符合条件的职工信息" << endl;
}
}
// 统计各科室平均工资
void calculateAverageSalary() {
cout << "各科室平均工资如下:" << endl;
double totalSalary = 0.0;
int employeeCount = 0;
string currentDepartment = "";
for (Employee& employee : employees) {
if (employee.department != currentDepartment) {
if (employeeCount > 0) {
double averageSalary = totalSalary / employeeCount;
cout << currentDepartment << ": " << averageSalary << endl;
}
totalSalary = 0.0;
employeeCount = 0;
currentDepartment = employee.department;
}
totalSalary += employee.salary;
employeeCount++;
}
if (employeeCount > 0) {
double averageSalary = totalSalary / employeeCount;
cout << currentDepartment << ": " << averageSalary << endl;
}
}
// 按工资从小到大排序输出
void sortBySalary() {
sort(employees.begin(), employees.end());
for (Employee& employee : employees) {
cout << employee;
}
}
// 修改职工信息
void updateEmployee() {
int id;
cout << "请输入要修改的职工工号:";
cin >> id;
for (Employee& employee : employees) {
if (employee.id == id) {
cout << "请输入新的职工信息(姓名 性别 工号 电话 科室 工资):";
cin >> employee;
cout << "职工信息已更新" << endl;
saveToFile();
return;
}
}
cout << "未找到该职工信息" << endl;
}
// 删除职工信息
void deleteEmployee() {
int id;
cout << "请输入要删除的职工工号:";
cin >> id;
for (auto it = employees.begin(); it != employees.end(); it++) {
if (it->id == id) {
employees.erase(it);
cout << "职工信息已删除" << endl;
saveToFile();
return;
}
}
cout << "未找到该职工信息" << endl;
}
};
int main() {
EmployeeManager manager;
while (true) {
manager.showMenu();
int choice;
cin >> choice;
switch (choice) {
case 1:
manager.addEmployee();
break;
case 2:
manager.exactQueryEmployee();
break;
case 3:
manager.fuzzyQueryEmployee();
break;
case 4:
manager.calculateAverageSalary();
break;
case 5:
manager.sortBySalary();
break;
case 6:
manager.updateEmployee();
break;
case 7:
manager.deleteEmployee();
break;
case 8:
return 0;
default:
cout << "无效的选择,请重新输入" << endl;
}
}
return 0;
}
```
使用方法:
1. 将以上代码保存为`employee.cpp`
2. 编译代码:`g++ employee.cpp -o employee`
3. 运行程序:`./employee`
注意事项:
1. 程序会在当前目录下创建一个`employees.txt`文件来保存职工信息。
2. 请按照程序要求输入职工信息,否则程序可能会出错。
3. 程序没有做输入检查,如果输入不合法可能会导致程序崩溃。
阅读全文
相关推荐
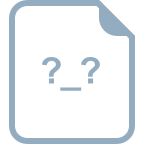
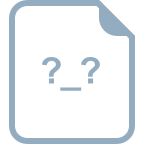
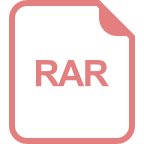









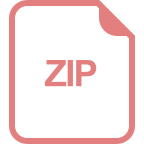
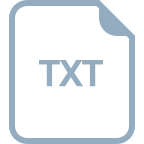
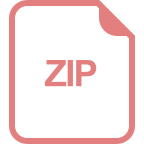
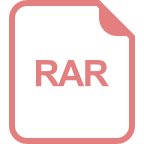
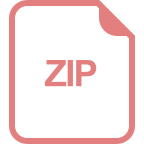
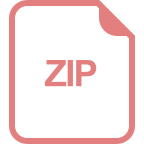
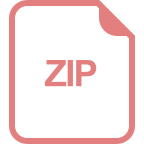