.net mvc中如何实现jwt认证
时间: 2023-07-18 07:07:10 浏览: 57
在 ASP.NET MVC 中实现 JWT 认证需要以下步骤:
1. 安装 `Microsoft.AspNetCore.Authentication.JwtBearer` 包
可以通过 NuGet 包管理器或者命令行安装该包。
2. 在 `Startup.cs` 文件中添加认证服务
在 `ConfigureServices` 方法中添加如下代码:
```csharp
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = "your_issuer",
ValidAudience = "your_audience",
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes("your_secret_key"))
};
});
```
其中,`your_issuer` 和 `your_audience` 分别代表你的应用程序的发行者和接收者,`your_secret_key` 是用于签名和验证 JWT 的密钥。
3. 在 `Configure` 方法中启用认证服务
在 `Configure` 方法中添加如下代码:
```csharp
app.UseAuthentication();
```
4. 在控制器中添加 `[Authorize]` 属性
在需要进行认证的控制器或者方法上添加 `[Authorize]` 属性即可。
5. 在登录时生成 JWT 并将其返回给客户端
在登录验证成功后,生成 JWT 并将其返回给客户端。可以使用 `System.IdentityModel.Tokens.Jwt` 包来生成 JWT,示例代码如下:
```csharp
var tokenHandler = new JwtSecurityTokenHandler();
var key = Encoding.UTF8.GetBytes("your_secret_key");
var tokenDescriptor = new SecurityTokenDescriptor
{
Subject = new ClaimsIdentity(new Claim[]
{
new Claim(ClaimTypes.Name, "your_username"),
new Claim(ClaimTypes.Role, "your_role")
}),
Expires = DateTime.UtcNow.AddDays(7),
SigningCredentials = new SigningCredentials(new SymmetricSecurityKey(key), SecurityAlgorithms.HmacSha256Signature)
};
var token = tokenHandler.CreateToken(tokenDescriptor);
var jwt = tokenHandler.WriteToken(token);
```
其中,`your_username` 和 `your_role` 分别代表该用户的用户名和角色。
以上就是在 ASP.NET MVC 中实现 JWT 认证的基本步骤。
相关推荐
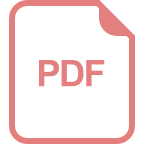
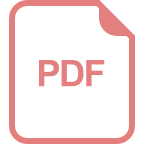
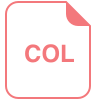
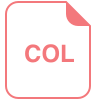
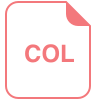
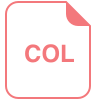










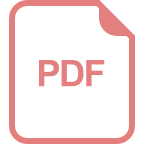