利用OpenCV及数字图片模板匹配法实现一个银行卡号检测并提取出来
时间: 2024-04-05 14:34:47 浏览: 50
好的,我可以为您提供一些思路和代码示例。
首先,您需要安装OpenCV库并了解数字图片模板匹配法的基本原理。数字图片模板匹配法是将一个小的模板图像与一幅大图像进行匹配,从而找到模板在大图像中的位置。在这种情况下,模板图像是数字字符,大图像是银行卡号的图像。
以下是实现银行卡号检测的大致步骤:
1. 读取银行卡号的图像并将其转换为灰度图像。
```
import cv2
import numpy as np
img = cv2.imread('bank_card.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 准备数字字符模板。您可以使用任何数字字符图像,将其存储到文件夹中,例如数字0到9的图片文件。
```
templates = []
for i in range(10):
template = cv2.imread('template{}.jpg'.format(i), 0)
templates.append(template)
```
3. 对每个数字字符模板进行模板匹配,并提取匹配结果。
```
results = []
for template in templates:
result = cv2.matchTemplate(gray, template, cv2.TM_CCOEFF_NORMED)
results.append(result)
```
4. 根据匹配结果,找到每个数字字符在银行卡号图像中的位置。
```
locations = []
for result in results:
location = np.where(result >= 0.8)
locations.append(location)
```
5. 将找到的数字字符位置拼接成银行卡号。
```
card_number = ''
for location in locations:
for pt in zip(*location[::-1]):
card_number += str(img[pt[1]:pt[1]+template.shape[1], pt[0]:pt[0]+template.shape[0]])
```
完整代码示例如下:
```
import cv2
import numpy as np
img = cv2.imread('bank_card.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
templates = []
for i in range(10):
template = cv2.imread('template{}.jpg'.format(i), 0)
templates.append(template)
results = []
for template in templates:
result = cv2.matchTemplate(gray, template, cv2.TM_CCOEFF_NORMED)
results.append(result)
locations = []
for result in results:
location = np.where(result >= 0.8)
locations.append(location)
card_number = ''
for location in locations:
for pt in zip(*location[::-1]):
card_number += str(img[pt[1]:pt[1]+template.shape[1], pt[0]:pt[0]+template.shape[0]])
print(card_number)
```
需要注意的是,以上示例代码仅适用于数字字符与银行卡号图像颜色相同的情况。如果数字字符与银行卡号图像颜色不同,您需要在处理之前对银行卡号图像进行颜色空间转换,例如从BGR转换到HSV,并使用颜色分割技术将数字字符分割出来。
阅读全文
相关推荐
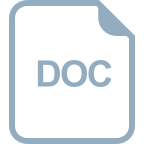
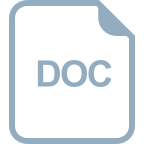
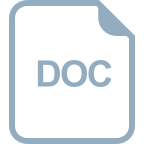


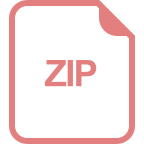
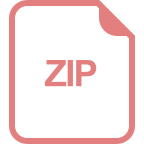
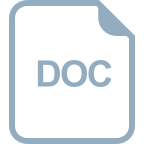
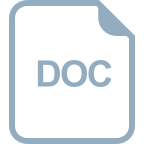
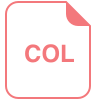
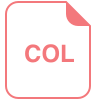
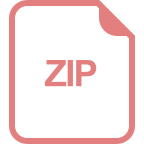
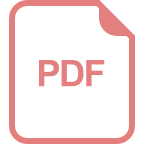
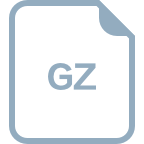
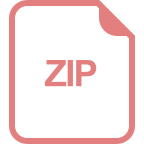
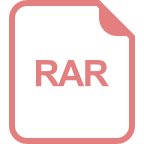
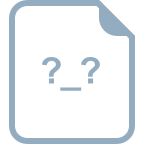
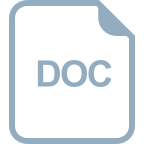
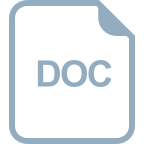