RuntimeError: median() operation does not have an identity for empty input tensor
时间: 2024-02-15 12:31:12 浏览: 20
This error occurs when you try to calculate the median of an empty tensor in PyTorch. The median is defined as the middle value of a sorted list of numbers. If the tensor is empty, there are no values to sort and find the middle value, hence an error is raised.
To fix this error, you need to check if the tensor is empty before computing the median. You can do this using the `torch.numel()` function which returns the number of elements in the tensor. If the tensor is empty, you can either return a default value or raise an exception.
Here's an example of how to handle this error:
```
import torch
# create an empty tensor
x = torch.tensor([])
# check if the tensor is empty
if torch.numel(x) == 0:
# handle empty tensor
print("Tensor is empty!")
else:
# compute median
median = torch.median(x)
print("Median:", median)
```
In this example, we first create an empty tensor `x`. We then check if the tensor is empty using `torch.numel()`. If the tensor is empty, we print a message saying that the tensor is empty. If the tensor is not empty, we compute the median using `torch.median()` and print the result.
By handling the case of an empty tensor, you can avoid the `RuntimeError` and ensure that your code runs smoothly.
相关推荐
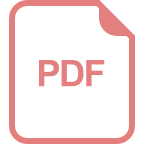
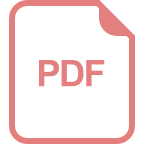
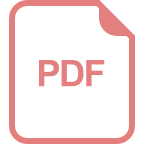













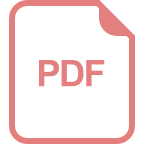
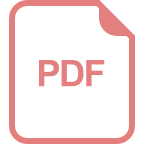