用c语言写一个简单的迷宫游戏
时间: 2023-10-19 14:05:09 浏览: 78
好的,下面是一个简单的迷宫游戏的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define ROWS 10
#define COLS 10
// 定义迷宫中的状态
enum Status {
WALL, // 墙
WAY, // 通路
VISITED // 访问过的通路
};
// 定义方向
enum Direction {
UP,
DOWN,
LEFT,
RIGHT
};
// 初始化迷宫
void init_maze(enum Status maze[][COLS]) {
// 将所有格子都初始化为墙
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
maze[i][j] = WALL;
}
}
// 生成随机起点和终点,起点为(0, 0),终点为(ROWS-1, COLS-1)
srand(time(NULL));
maze[0][0] = WAY;
maze[ROWS-1][COLS-1] = WAY;
// 生成随机的通路
int row, col;
for (int i = 0; i < ROWS * COLS / 3; i++) {
row = rand() % (ROWS - 2) + 1;
col = rand() % (COLS - 2) + 1;
maze[row][col] = WAY;
}
}
// 打印迷宫
void print_maze(enum Status maze[][COLS]) {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (maze[i][j] == WALL) {
printf("#");
} else if (maze[i][j] == WAY) {
printf(" ");
} else if (maze[i][j] == VISITED) {
printf(".");
}
}
printf("\n");
}
}
// 判断某个格子是否可以访问
int can_visit(enum Status maze[][COLS], int row, int col) {
if (row < 0 || row >= ROWS || col < 0 || col >= COLS) {
return 0;
}
if (maze[row][col] == WAY || maze[row][col] == VISITED) {
return 0;
}
return 1;
}
// 按指定方向移动
void move(enum Direction direction, int *row, int *col) {
if (direction == UP) {
(*row)--;
} else if (direction == DOWN) {
(*row)++;
} else if (direction == LEFT) {
(*col)--;
} else if (direction == RIGHT) {
(*col)++;
}
}
// 随机选择下一步的方向
enum Direction choose_direction() {
int rand_num = rand() % 4;
return (enum Direction) rand_num;
}
// 递归地生成迷宫
void generate_maze(enum Status maze[][COLS], int row, int col) {
maze[row][col] = VISITED;
while (1) {
enum Direction direction = choose_direction();
move(direction, &row, &col);
if (can_visit(maze, row, col)) {
maze[row][col] = VISITED;
generate_maze(maze, row, col);
} else {
move(direction + 2, &row, &col); // 返回上一步
}
if (row == 0 && col == 0) { // 如果回到了起点,表示迷宫已生成
break;
}
}
}
int main() {
enum Status maze[ROWS][COLS];
init_maze(maze);
generate_maze(maze, 0, 0);
print_maze(maze);
return 0;
}
```
这个迷宫游戏使用递归深度优先搜索算法生成迷宫,然后打印出来。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐




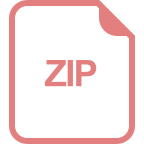

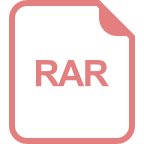
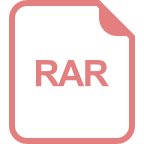






