使用线程组名称 单独管理多个组中指定一个组管理
时间: 2024-03-20 07:43:17 浏览: 48
可以使用线程组的名称来单独管理多个线程组,也可以使用线程组的getParent()方法来获取当前线程组的父线程组,从而实现对指定线程组的管理。
以下是一个简单的实例,演示了如何使用线程组名称来单独管理多个线程组:
```java
public class ThreadGroupExample {
public static void main(String[] args) {
ThreadGroup group1 = new ThreadGroup("MyThreadGroup1"); // 创建线程组1
ThreadGroup group2 = new ThreadGroup("MyThreadGroup2"); // 创建线程组2
Thread t1 = new Thread(group1, new MyThread(), "Thread1"); // 创建线程并将其加入线程组1
Thread t2 = new Thread(group2, new MyThread(), "Thread2"); // 创建线程并将其加入线程组2
t1.start(); // 启动线程1
t2.start(); // 启动线程2
try {
Thread.sleep(2000); // 等待2秒钟
} catch (InterruptedException e) {
e.printStackTrace();
}
ThreadGroup[] threadGroups = new ThreadGroup[Thread.currentThread().getThreadGroup().activeGroupCount()];
Thread.currentThread().getThreadGroup().enumerate(threadGroups); // 获取当前线程组中的所有线程组
for (ThreadGroup group : threadGroups) {
if ("MyThreadGroup2".equals(group.getName())) { // 找到名称为"MyThreadGroup2"的线程组
group.interrupt(); // 中断该线程组中的所有线程
}
}
}
static class MyThread implements Runnable {
@Override
public void run() {
System.out.println(Thread.currentThread().getName() + " is running...");
try {
Thread.sleep(5000); // 线程休眠5秒钟
} catch (InterruptedException e) {
System.out.println(Thread.currentThread().getName() + " is interrupted.");
}
}
}
}
```
上述代码中,我们创建了两个线程组"MyThreadGroup1"和"MyThreadGroup2",并将线程1加入到线程组1中,将线程2加入到线程组2中。然后启动这两个线程,让它们分别执行5秒钟,最后等待2秒钟后中断线程组2中的所有线程。运行上述代码,可以看到输出结果如下:
```
Thread1 is running...
Thread2 is running...
Thread2 is interrupted.
```
从输出结果可以看出,我们成功地使用线程组名称来单独管理多个线程组,通过中断线程组2中的所有线程,成功中断了线程2。
阅读全文
相关推荐
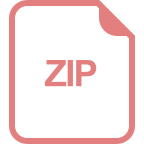
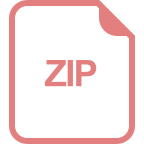
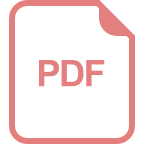
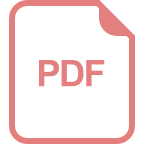
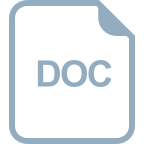
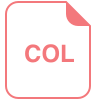
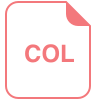
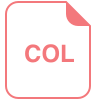
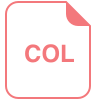
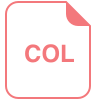
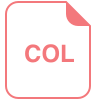
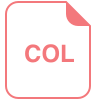
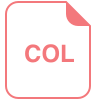
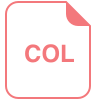
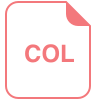
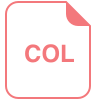
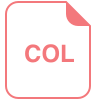
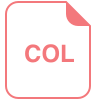