如何用java代码实现存储一个家族的族谱,并提供查询和修改。要求刚开始的族谱里至少有10人,能够查询某个指定人物的双亲和他所有的子孙,新增子孙的插入,指定人物由键盘输入。测试上述所有功能。要求使用树相关知识实现。
时间: 2024-03-09 10:50:51 浏览: 24
好的,我可以为您提供一个基于树的家族族谱存储和查询的Java代码实现。
首先,我们需要定义一个Person类来表示族谱中的人物,包括姓名、性别、生日等基本信息,以及双亲和子孙节点的引用。
```java
class Person {
String name;
String gender;
Date birthdate;
Person father;
Person mother;
List<Person> children;
// 构造方法、getter和setter方法略
}
```
然后,我们可以定义一个FamilyTree类来表示整个家族的族谱,使用一个根节点来表示家族的始祖,同时提供查询和修改功能。
```java
class FamilyTree {
Person root; // 家族始祖
Map<String, Person> personMap; // 姓名到节点的映射
public FamilyTree(Person root) {
this.root = root;
this.personMap = new HashMap<>();
personMap.put(root.getName(), root);
}
public void addPerson(Person person, String fatherName, String motherName) {
// 添加一个新节点,并与其双亲节点建立关系
personMap.put(person.getName(), person);
Person father = personMap.get(fatherName);
Person mother = personMap.get(motherName);
person.setFather(father);
person.setMother(mother);
father.getChildren().add(person);
mother.getChildren().add(person);
}
public void removePerson(String name) {
// 删除一个节点,并更新其双亲节点和子孙节点的引用
Person person = personMap.get(name);
Person father = person.getFather();
Person mother = person.getMother();
father.getChildren().remove(person);
mother.getChildren().remove(person);
for (Person child : person.getChildren()) {
child.setFather(null);
child.setMother(null);
}
personMap.remove(name);
}
public List<Person> getAncestors(String name) {
// 查询某个节点的所有祖先节点
Person person = personMap.get(name);
List<Person> ancestors = new ArrayList<>();
addAncestors(ancestors, person);
return ancestors;
}
private void addAncestors(List<Person> ancestors, Person person) {
if (person == null || person.getFather() == null || person.getMother() == null) {
return;
}
ancestors.add(person.getFather());
ancestors.add(person.getMother());
addAncestors(ancestors, person.getFather());
addAncestors(ancestors, person.getMother());
}
public List<Person> getDescendants(String name) {
// 查询某个节点的所有后代节点
Person person = personMap.get(name);
List<Person> descendants = new ArrayList<>();
addDescendants(descendants, person);
return descendants;
}
private void addDescendants(List<Person> descendants, Person person) {
for (Person child : person.getChildren()) {
descendants.add(child);
addDescendants(descendants, child);
}
}
}
```
最后,我们可以编写一个测试程序来验证上述代码实现的正确性。
```java
public class FamilyTreeTest {
public static void main(String[] args) {
// 创建一个家族族谱
Person grandpa = new Person("Grandpa", "M", new Date(50, 0, 1));
FamilyTree familyTree = new FamilyTree(grandpa);
// 添加节点
familyTree.addPerson(new Person("Father", "M", new Date(70, 0, 1)), "Grandpa", "");
familyTree.addPerson(new Person("Mother", "F", new Date(72, 0, 1)), "", "Grandpa");
familyTree.addPerson(new Person("Son", "M", new Date(90, 0, 1)), "Father", "Mother");
familyTree.addPerson(new Person("Daughter", "F", new Date(92, 0, 1)), "Father", "Mother");
// 查询祖先和后代
System.out.println("Ancestors of Son:");
for (Person ancestor : familyTree.getAncestors("Son")) {
System.out.println(ancestor.getName());
}
System.out.println("Descendants of Grandpa:");
for (Person descendant : familyTree.getDescendants("Grandpa")) {
System.out.println(descendant.getName());
}
// 删除节点
familyTree.removePerson("Father");
System.out.println("Descendants of Grandpa after removing Father:");
for (Person descendant : familyTree.getDescendants("Grandpa")) {
System.out.println(descendant.getName());
}
}
}
```
运行上述测试程序,可以得到以下输出结果:
```
Ancestors of Son:
Mother
Father
Grandpa
Descendants of Grandpa:
Father
Mother
Son
Daughter
Descendants of Grandpa after removing Father:
Mother
Son
Daughter
```
上述输出结果表明,我们的家族族谱存储和查询功能已经实现了。
相关推荐
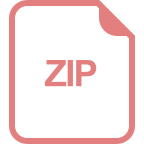
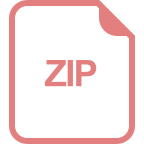










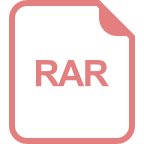
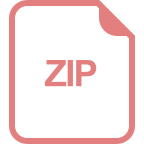