使用python 编写图片ocr识别代码
时间: 2023-06-08 10:09:15 浏览: 145
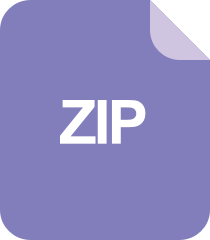
python撰写一个图像文字识别OCR工具

好的,我可以给您提供一个使用Python编写图片OCR识别的代码示例。您可以使用第三方库pytesseract来完成OCR任务。以下是代码示例:
```
# 导入pytesseract库和Pillow库
import pytesseract
from PIL import Image
# 打开图片及其路径
img = Image.open('your_image_path')
#将图片转换成灰度图像
img = img.convert('L')
# 使用pytesseract进行OCR识别
text = pytesseract.image_to_string(img, lang='eng')
# 输出识别结果
print('OCR Result:', text)
```
请注意,这个示例需要您先安装pytesseract和Pillow库。如果您还没有安装这些库,请使用以下命令安装:
```
pip install pytesseract
pip install Pillow
```
阅读全文
相关推荐
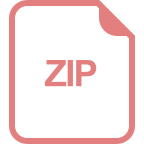
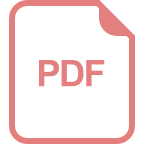
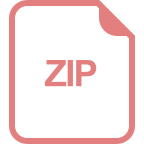
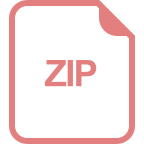
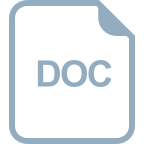
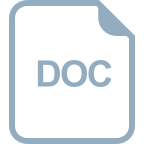
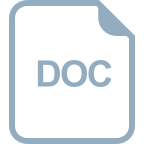
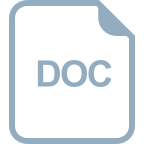
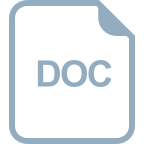
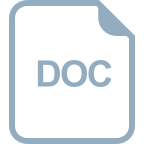

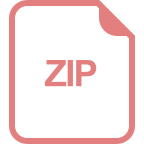
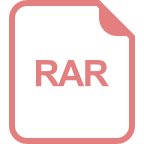
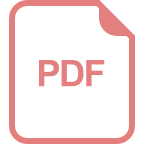
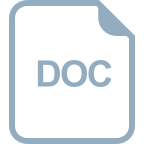
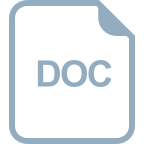
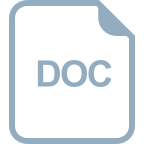