请设计周期置换密码加解密的python实现程序
时间: 2024-05-01 09:20:57 浏览: 82
周期置换密码是一种简单的加密算法,它通过对明文进行周期性的置换来实现加密,具体步骤如下:
1. 将明文分成若干个长度为n的块,最后一个块如果不足n个字符,则在末尾补足相应的字符。
2. 定义一个长度为n的置换表,将每个块按照置换表进行置换。
3. 将置换后的块连接起来,得到密文。
解密的过程与加密相反,只需要将置换表反向使用即可。
下面是一个简单的Python实现:
```python
def encrypt(plain_text, key):
n = len(key)
cipher_text = ''
for i in range(0, len(plain_text), n):
block = plain_text[i:i+n]
if len(block) < n:
block += ' ' * (n - len(block))
permuted_block = ''
for j in range(n):
permuted_block += block[key[j]-1]
cipher_text += permuted_block
return cipher_text
def decrypt(cipher_text, key):
n = len(key)
plain_text = ''
for i in range(0, len(cipher_text), n):
block = cipher_text[i:i+n]
permuted_block = ''
for j in range(n):
permuted_block += block[key.index(j+1)]
plain_text += permuted_block
return plain_text.strip()
# 示例
plain_text = 'hello world'
key = [5, 2, 1, 3, 4]
cipher_text = encrypt(plain_text, key)
print(cipher_text) # 'oelhlw lrod'
decrypted_text = decrypt(cipher_text, key)
print(decrypted_text) # 'hello world'
```
在上面的示例中,我们使用了一个长度为5的置换表 `[5, 2, 1, 3, 4]`,对明文进行了加密和解密。加密后的密文为 `'oelhlw lrod'`,解密后得到的明文为 `'hello world'`。
阅读全文
相关推荐
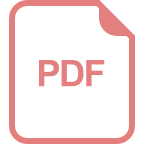
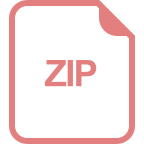

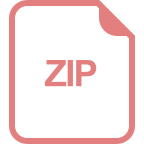
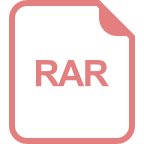
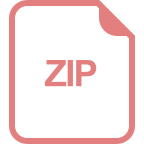
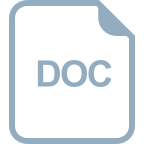
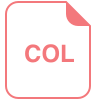
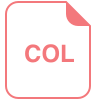
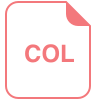
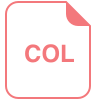
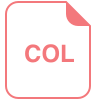
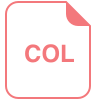
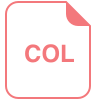
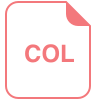
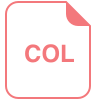
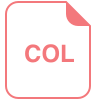
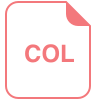