js获取本月当前周之前的所有日期
时间: 2023-10-25 16:08:04 浏览: 88
可以使用Date对象中的方法来实现,具体代码如下:
```
var now = new Date(); // 获取当前时间
var year = now.getFullYear(); // 获取当前年份
var month = now.getMonth() + 1; // 获取当前月份,需要加1
var dates = []; // 存储结果的数组
// 循环遍历当前月份之前的所有日期
for (var i = 1; i <= now.getDate(); i++) {
var date = new Date(year, month - 1, i); // 初始化为当前年月日,注意月份需要减1
if (date.getDay() === 0) { // 判断是否为周日
dates.push(date.getFullYear() + "-" + (date.getMonth() + 1) + "-" + date.getDate()); // 添加到结果数组中
}
}
console.log(dates); // 输出结果
```
当然,也可以根据需要,修改代码逻辑,获取其他时间段之前的所有日期。
相关问题
js获取本月截止到当前周的所有日期
可以使用以下代码获取本月截止到当前周的所有日期:
```javascript
var today = new Date(); // 获取当前日期
var firstDay = new Date(today.getFullYear(), today.getMonth(), 1); // 获取本月第一天日期
var currentWeekDay = today.getDay(); // 获取当前星期几
var endDay = new Date(today.getFullYear(), today.getMonth() + 1, 0); // 获取本月最后一天日期
var currentWeekEnd = new Date(today.getFullYear(), today.getMonth(), today.getDate() + (6 - currentWeekDay)); // 获取本周的周末日期
var currentWeekDates = []; // 存储本月截止到当前周的所有日期
for (var i = firstDay.getDate(); i <= currentWeekEnd.getDate(); i++) {
currentWeekDates.push(new Date(today.getFullYear(), today.getMonth(), i));
}
```
这段代码会返回一个数组 `currentWeekDates`,包含本月截止到当前周的所有日期。
js获取日期本周之前的日期
可以使用JavaScript的Date对象,结合setDate()方法和getTime()方法来获取本周之前的日期。具体实现方式可以参考以下代码:
```javascript
var now = new Date(); //获取当前时间
var days = now.getDay(); //获取当前时间是本周的第几天
var milliseconds = now.getTime() - days * 24 * 60 * 60 * 1000; //计算本周的第一天的时间戳
var lastWeek = new Date(milliseconds); //将时间戳转换为日期对象
var year = lastWeek.getFullYear(); //获取年份
var month = lastWeek.getMonth() + 1; //获取月份,需要+1
var date = lastWeek.getDate(); //获取日期
console.log("本周的第一天是:" + year + "-" + month + "-" + date); //输出本周的第一天
```
当然,也可以使用第三方库如Moment.js来简化代码。
阅读全文
相关推荐
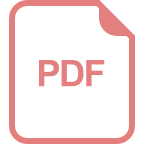
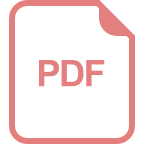





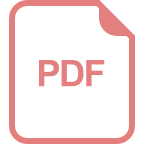
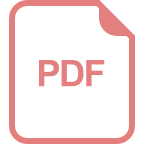
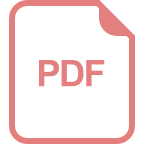





