遗传算法求函数最值(C语言实现)
时间: 2023-11-26 12:06:28 浏览: 127
好的,以下是一个遗传算法求函数最值的 C 语言实现代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <math.h>
// 遗传算法相关参数
#define POP_SIZE 100 // 种群大小
#define GEN_NUM 100 // 迭代次数
#define CROSSOVER_RATE 0.8 // 交叉概率
#define MUTATION_RATE 0.01 // 变异概率
#define ELITISM 1 // 精英选择
// 函数相关参数
#define X_LOWER_BOUND -10 // 自变量下界
#define X_UPPER_BOUND 10 // 自变量上界
// 目标函数
double target_func(double x) {
return sin(x) * x;
}
// 随机生成一个染色体
double* generate_chromosome() {
double* chromosome = (double*)malloc(sizeof(double));
chromosome[0] = X_LOWER_BOUND + (double)rand() / RAND_MAX * (X_UPPER_BOUND - X_LOWER_BOUND);
return chromosome;
}
// 计算染色体的适应度
double calculate_fitness(double* chromosome) {
double x = chromosome[0];
return target_func(x);
}
// 选择操作
void selection(double** population, double** new_population) {
int i, j;
double fitness_sum = 0.0;
double* fitness = (double*)malloc(sizeof(double) * POP_SIZE);
// 计算所有染色体的适应度总和
for (i = 0; i < POP_SIZE; ++i) {
fitness[i] = calculate_fitness(population[i]);
fitness_sum += fitness[i];
}
// 轮盘赌选择
for (i = 0; i < POP_SIZE; ++i) {
double rand_num = (double)rand() / RAND_MAX * fitness_sum;
double cumulative_fitness = 0.0;
for (j = 0; j < POP_SIZE; ++j) {
cumulative_fitness += fitness[j];
if (rand_num <= cumulative_fitness) {
new_population[i][0] = population[j][0];
break;
}
}
}
free(fitness);
}
// 交叉操作
void crossover(double** population, double** new_population) {
int i, j;
for (i = 0; i < POP_SIZE; i += 2) {
double rand_num = (double)rand() / RAND_MAX;
if (rand_num < CROSSOVER_RATE) {
int crossover_point = rand() % (sizeof(double) * 8);
double temp = population[i][0];
population[i][0] = (population[i][0] & ((-1 << crossover_point))) | (population[i + 1][0] & ((1 << crossover_point) - 1));
population[i + 1][0] = (population[i + 1][0] & ((1 << crossover_point) - 1)) | (temp & ((-1 << crossover_point)));
}
new_population[i][0] = population[i][0];
new_population[i + 1][0] = population[i + 1][0];
}
}
// 变异操作
void mutation(double** population, double** new_population) {
int i, j;
for (i = 0; i < POP_SIZE; ++i) {
double rand_num = (double)rand() / RAND_MAX;
if (rand_num < MUTATION_RATE) {
int mutation_point = rand() % (sizeof(double) * 8);
population[i][0] = population[i][0] ^ (1 << mutation_point);
}
new_population[i][0] = population[i][0];
}
}
// 精英选择
void elitism(double** population, double** new_population) {
int i, j;
int best_chromosome_index = 0;
double best_fitness = calculate_fitness(population[0]);
// 找到当前种群中适应度最好的染色体
for (i = 1; i < POP_SIZE; ++i) {
double fitness = calculate_fitness(population[i]);
if (fitness > best_fitness) {
best_chromosome_index = i;
best_fitness = fitness;
}
}
// 将最好的染色体复制到下一代种群中
for (i = 0; i < ELITISM; ++i) {
new_population[i][0] = population[best_chromosome_index][0];
}
}
int main() {
int i, j;
double** population = (double**)malloc(sizeof(double*) * POP_SIZE);
double** new_population = (double**)malloc(sizeof(double*) * POP_SIZE);
// 随机初始化种群
for (i = 0; i < POP_SIZE; ++i) {
population[i] = generate_chromosome();
new_population[i] = (double*)malloc(sizeof(double));
}
// 迭代
for (i = 0; i < GEN_NUM; ++i) {
selection(population, new_population);
crossover(population, new_population);
mutation(population, new_population);
elitism(population, new_population);
// 将下一代种群复制回当前种群
for (j = 0; j < POP_SIZE; ++j) {
population[j][0] = new_population[j][0];
}
}
// 找到适应度最好的染色体
int best_chromosome_index = 0;
double best_fitness = calculate_fitness(population[0]);
for (i = 1; i < POP_SIZE; ++i) {
double fitness = calculate_fitness(population[i]);
if (fitness > best_fitness) {
best_chromosome_index = i;
best_fitness = fitness;
}
}
// 输出结果
printf("x = %lf, f(x) = %lf\n", population[best_chromosome_index][0], best_fitness);
// 释放内存
for (i = 0; i < POP_SIZE; ++i) {
free(population[i]);
free(new_population[i]);
}
free(population);
free(new_population);
return 0;
}
```
该示例代码实现了一个简单的遗传算法,用于求解一个函数的最大值。通过多次迭代,遗传算法能够不断优化种群,逐渐接近函数的最大值。在本例中,我们以 $y = \sin(x) \times x$ 为目标函数,对 $x$ 进行优化,其中 $x$ 的范围为 $[-10, 10]$。在初始化种群后,算法进行一系列选择、交叉和变异操作,最终得到适应度最好的染色体,即对应的 $x$ 值,即为函数的最大值点。
阅读全文
相关推荐

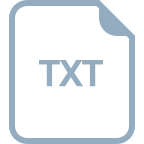
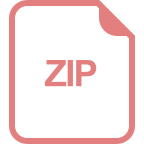
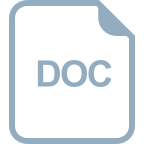
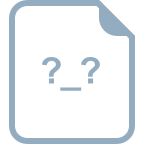
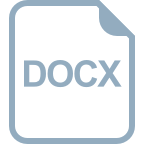
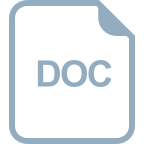
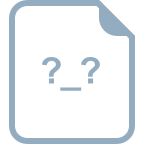
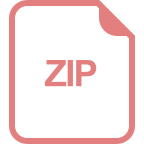
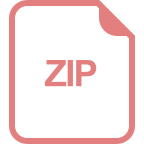
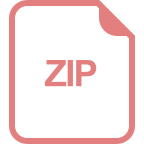