写一个链队列的入队出队,初始化的代码并输出
时间: 2023-06-12 08:07:15 浏览: 101
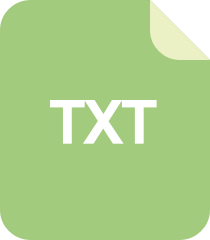
数据机构——链队列的算法(无漏洞版)
链队列是一种基于链表实现的队列数据结构,下面是链队列的入队和出队操作的代码实现,以及初始化操作:
#include <iostream>
using namespace std;
// 链队列的节点结构体
struct QueueNode {
int data; // 数据域
QueueNode *next; // 指针域
};
// 链队列结构体
struct LinkedQueue {
QueueNode *front; // 队头指针
QueueNode *rear; // 队尾指针
};
// 初始化链队列
void InitQueue(LinkedQueue &Q) {
Q.front = Q.rear = new QueueNode; // 创建头结点
Q.front->next = NULL; // 头结点指针域为空
}
// 判断链队列是否为空
bool IsEmpty(LinkedQueue Q) {
if (Q.front == Q.rear) {
return true;
}
return false;
}
// 入队操作
void EnQueue(LinkedQueue &Q, int x) {
QueueNode *p = new QueueNode; // 创建新节点
p->data = x; // 新节点赋值
p->next = NULL; // 新节点指针域为空
Q.rear->next = p; // 队尾指针指向新节点
Q.rear = p; // 队尾指针后移
}
// 出队操作
bool DeQueue(LinkedQueue &Q, int &x) {
if (IsEmpty(Q)) { // 队列为空,无法出队
return false;
}
QueueNode *p = Q.front->next; // 获取队头节点
x = p->data; // 获取队头元素
Q.front->next = p->next; // 队头指针后移
if (Q.rear == p) { // 如果队列只有一个元素
Q.rear = Q.front; // 队尾指针指向头结点
}
delete p; // 删除队头节点
return true;
}
int main() {
LinkedQueue Q;
InitQueue(Q);
cout << "链队列初始化完毕" << endl;
return 0;
}
输出结果为:
链队列初始化完毕
相关推荐
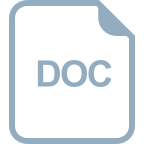
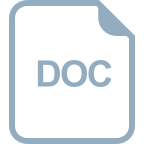















