用Java 写段 RSA 加密算法
时间: 2023-08-21 11:27:55 浏览: 182
以下是使用Java实现RSA加密算法的示例代码:
```java
import java.math.BigInteger;
import java.util.Random;
public class RSA {
private BigInteger n, d, e;
private int bitlen = 1024;
public RSA() {
Random r = new Random();
BigInteger p = BigInteger.probablePrime(bitlen / 2, r);
BigInteger q = BigInteger.probablePrime(bitlen / 2, r);
n = p.multiply(q);
BigInteger m = (p.subtract(BigInteger.ONE)).multiply(q.subtract(BigInteger.ONE));
e = BigInteger.probablePrime(bitlen / 2, r);
while (m.gcd(e).intValue() > 1) {
e = BigInteger.probablePrime(bitlen / 2, r);
}
d = e.modInverse(m);
}
public RSA(BigInteger e, BigInteger d, BigInteger n) {
this.e = e;
this.d = d;
this.n = n;
}
public synchronized BigInteger[] encrypt(byte[] message) {
BigInteger[] cipher = new BigInteger[message.length];
for (int i = 0; i < message.length; i++) {
cipher[i] = BigInteger.valueOf(message[i]).modPow(e, n);
}
return cipher;
}
public synchronized byte[] decrypt(BigInteger[] cipher) {
byte[] message = new byte[cipher.length];
for (int i = 0; i < message.length; i++) {
message[i] = cipher[i].modPow(d, n).byteValue();
}
return message;
}
public static void main(String[] args) {
RSA rsa = new RSA();
String text1 = "Hello World";
System.out.println("Original Text 1: " + text1);
//加密
byte[] plaintext1 = text1.getBytes();
BigInteger[] ciphertext1 = rsa.encrypt(plaintext1);
System.out.print("Ciphertext 1: ");
for (int i = 0; i < ciphertext1.length; i++) {
System.out.print(ciphertext1[i].toString() + " ");
}
System.out.println();
//解密
byte[] decrypted1 = rsa.decrypt(ciphertext1);
System.out.println("Decrypted Text 1: " + new String(decrypted1));
String text2 = "RSA is an asymmetric cryptographic algorithm";
System.out.println("Original Text 2: " + text2);
//加密
byte[] plaintext2 = text2.getBytes();
BigInteger[] ciphertext2 = rsa.encrypt(plaintext2);
System.out.print("Ciphertext 2: ");
for (int i = 0; i < ciphertext2.length; i++) {
System.out.print(ciphertext2[i].toString() + " ");
}
System.out.println();
//解密
byte[] decrypted2 = rsa.decrypt(ciphertext2);
System.out.println("Decrypted Text 2: " + new String(decrypted2));
}
}
```
上述代码实现了一个简单的RSA加密算法,包括密钥生成、加密和解密功能。在main函数中,我们加密了两个字符串并进行了解密。
阅读全文
相关推荐
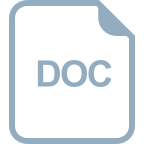
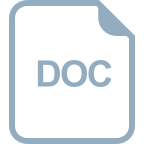
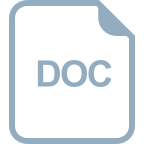
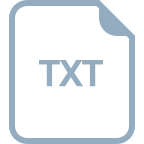
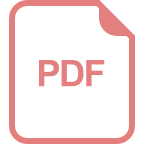
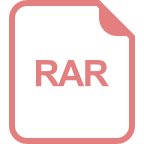
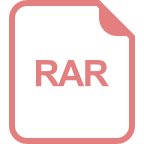
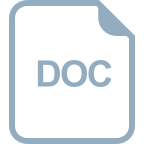
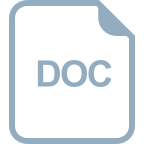
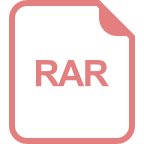
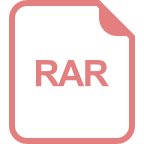
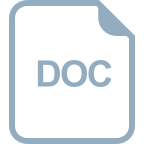
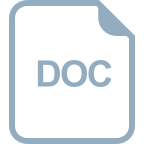
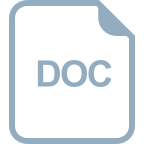
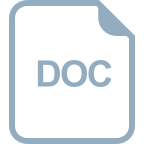
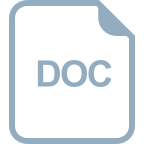
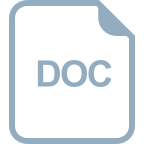