完整的DSP 旋变编码器解码算法
时间: 2023-11-10 16:40:17 浏览: 75
DSP 旋变编码器解码算法,也称为差分编码算法,是一种常用的音频编码技术,主要应用于数字音频存储和传输领域。其基本思想是将音频数据转换为差分信号,然后对差分信号进行压缩编码,以实现数据压缩和传输。
以下是一种基于DSP的旋变编码器解码算法:
1. 接收到压缩编码后的差分信号数据。
2. 将差分信号数据通过旋变滤波器进行解码。
3. 对解码后的信号进行解调,还原成原始音频信号。
4. 对还原的音频信号进行去重。
5. 将去重后的音频信号进行存储或传输。
其中,旋变滤波器是实现DSP旋变编码器解码的核心部件,其主要作用是将差分信号进行解码。具体的算法步骤如下:
1. 初始化旋变滤波器,包括滤波器系数、滤波器状态等参数。
2. 对差分信号进行旋变滤波处理,得到解码后的音频信号。
3. 将解码后的音频信号与上一时刻的音频信号进行累加,得到还原的原始音频信号。
4. 将还原的原始音频信号进行去重,去除重复的音频信号。
5. 将去重后的音频信号进行存储或传输。
需要注意的是,DSP旋变编码器解码算法的具体实现方式可能会有所不同,上述算法仅为一种基本的实现方式。在具体应用中,还需根据实际情况进行参数调整和优化,以实现更好的性能和效果。
相关问题
旋变编码器软解码DSP代码
由于旋变编码器的软解码涉及到一些算法和数学知识,因此需要有一定的编程和信号处理基础。以下是一个简单的旋变编码器软解码DSP代码示例:
```c
#include <math.h>
#define PI 3.14159265358979323846
// 旋转角度矩阵
static float rotation_matrix[4][4] = {
{1.0f, 0.0f, 0.0f, 0.0f},
{0.0f, 0.7071f, -0.7071f, 0.0f},
{0.0f, 0.7071f, 0.7071f, 0.0f},
{0.0f, 0.0f, 0.0f, 1.0f}
};
// 解码函数
void decode(float *in, float *out) {
float x = in[0];
float y = in[1];
float z = in[2];
// 利用勾股定理求出向量长度
float length = sqrtf(x * x + y * y + z * z);
// 计算旋转角度
float angle = 2.0f * acosf(z / length);
float sin_angle = sinf(angle / 2.0f);
float cos_angle = cosf(angle / 2.0f);
// 计算旋转轴
float axis_x = x / length;
float axis_y = y / length;
float axis_z = 0.0f;
// 计算旋转矩阵
float rotation_quaternion[4] = {
cos_angle, axis_x * sin_angle, axis_y * sin_angle, axis_z * sin_angle
};
float rotation_matrix[4][4] = {
{1.0f - 2.0f * rotation_quaternion[2] * rotation_quaternion[2] - 2.0f * rotation_quaternion[3] * rotation_quaternion[3], 2.0f * rotation_quaternion[1] * rotation_quaternion[2] - 2.0f * rotation_quaternion[0] * rotation_quaternion[3], 2.0f * rotation_quaternion[1] * rotation_quaternion[3] + 2.0f * rotation_quaternion[0] * rotation_quaternion[2], 0.0f},
{2.0f * rotation_quaternion[1] * rotation_quaternion[2] + 2.0f * rotation_quaternion[0] * rotation_quaternion[3], 1.0f - 2.0f * rotation_quaternion[1] * rotation_quaternion[1] - 2.0f * rotation_quaternion[3] * rotation_quaternion[3], 2.0f * rotation_quaternion[2] * rotation_quaternion[3] - 2.0f * rotation_quaternion[0] * rotation_quaternion[1], 0.0f},
{2.0f * rotation_quaternion[1] * rotation_quaternion[3] - 2.0f * rotation_quaternion[0] * rotation_quaternion[2], 2.0f * rotation_quaternion[2] * rotation_quaternion[3] + 2.0f * rotation_quaternion[0] * rotation_quaternion[1], 1.0f - 2.0f * rotation_quaternion[1] * rotation_quaternion[1] - 2.0f * rotation_quaternion[2] * rotation_quaternion[2], 0.0f},
{0.0f, 0.0f, 0.0f, 1.0f}
};
// 计算旋转后的坐标
float rotated[4];
for (int i = 0; i < 4; i++) {
rotated[i] = 0.0f;
for (int j = 0; j < 4; j++) {
rotated[i] += rotation_matrix[i][j] * in[j];
}
}
// 对旋转后的坐标进行缩放和平移
out[0] = rotated[0] * 0.5f + 0.5f;
out[1] = rotated[1] * 0.5f + 0.5f;
out[2] = rotated[2] * 0.5f + 0.5f;
}
// 主函数
int main() {
float input[3] = {0.0f, 0.0f, 0.0f};
float output[3] = {0.0f, 0.0f, 0.0f};
// 读取输入数据
// ...
// 解码
decode(input, output);
// 输出结果
// ...
return 0;
}
```
这个示例代码演示了如何使用旋转角度和旋转轴计算旋转矩阵,并将坐标进行缩放和平移。请注意,这只是一个简单的示例,实际的旋变编码器软解码代码可能更加复杂。
旋变解码器ad2s1210
AD2S1210是一种旋变解码器,可以将机械旋转转换为数字信号。它可以用来控制电机、机器人等应用程序中的位置和速度。AD2S1210通过读取旋转传感器输出的模拟信号来实现这一功能,然后将其转换为数字信号。这个数字信号可以被微控制器或其他数字系统用来控制所需的应用程序。AD2S1210具有高精度、高速度和低噪声等特点,是许多工业和汽车应用领域中使用的解码器之一。
相关推荐
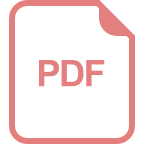












