c语言 解析正则表达式
时间: 2023-10-23 08:51:18 浏览: 118
C语言本身并没有内置解析正则表达式的功能,但是可以通过使用第三方库来实现。以下是一些常用的C语言正则表达式库:
1. PCRE(Perl Compatible Regular Expressions):这是一个流行的正则表达式库,支持Perl风格的正则表达式语法。它提供了C语言的API接口,可以轻松地在C程序中使用。
2. POSIX正则表达式库:这是一个基于POSIX标准的正则表达式库,也可以在C程序中使用。
3. TRE(Tab Regular Expressions):这是一个高效的正则表达式库,支持POSIX扩展正则表达式语法。它具有快速匹配速度和低内存消耗的优点。
4. Oniguruma:这是一个支持广泛的正则表达式语法的库,包括Perl、Ruby和JavaScript等语言的正则表达式语法。它也提供了C语言的API接口。
使用这些库的方法类似,一般需要先编译安装库文件,然后在C程序中引入相应的头文件,并使用库提供的API函数来操作正则表达式。具体的使用方法可以参考相应库的文档或示例代码。
相关问题
C语言 使用正则表达式 封装解析json工具类
可以使用C语言中的正则表达式库PCRE(Perl Compatible Regular Expressions)来解析JSON字符串。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <string.h>
#include <pcre.h>
#define OVECCOUNT 30
int parse_json(const char *json_str) {
int rc;
const char *error;
int erroffset;
int ovector[OVECCOUNT];
char key[128], value[128];
pcre *re = pcre_compile("\"([^\"]+)\": ?\"([^\"]+)\"", 0, &error, &erroffset, NULL);
if (!re) {
printf("pcre_compile failed (offset: %d), error message: %s\n", erroffset, error);
return -1;
}
const char *p = json_str;
while (*p) {
rc = pcre_exec(re, NULL, p, strlen(p), 0, 0, ovector, OVECCOUNT);
if (rc < 0) {
break;
}
pcre_copy_substring(json_str, ovector, rc, 1, key, sizeof(key));
pcre_copy_substring(json_str, ovector, rc, 2, value, sizeof(value));
printf("%s: %s\n", key, value);
p += ovector[1];
}
pcre_free(re);
return 0;
}
int main() {
const char *json_str = "{\"name\": \"John\", \"age\": 30}";
parse_json(json_str);
return 0;
}
```
上述代码使用PCRE库编译了一个正则表达式,用于匹配JSON字符串中的键值对,然后使用pcre_exec函数进行匹配。匹配成功后,使用pcre_copy_substring函数提取键和值,并输出到控制台。
C语言正则表达式匹配文件后缀名
在C语言中,正则表达式(Regular Expression)并不是原生支持的,标准库`<stdio.h>`和`<string.h>`提供的功能并不直接用于处理正则表达式。如果你需要在C语言中匹配文件后缀名,通常会使用第三方库,如PCRE(Perl Compatible Regular Expressions)或GRegex。
如果你想要使用正则表达式,可以先安装并链接相关的库,然后编写一个自定义函数来解析和匹配字符串。以下是一个简单的示例,展示如何使用PCRE库来匹配文件后缀:
```c
#include <stdio.h>
#include <pcre.h>
// 定义正则表达式
#define FILE_EXTENSION_REGEX "^.*\\.(\\w+)$"
// 匹配函数
int match_file_extension(const char *filename, const char *regex) {
int erroffset = 0;
pcre *compiled_regex = pcre_compile(regex, 0, &erroffset, NULL, NULL);
if (!compiled_regex) {
// 处理编译错误
fprintf(stderr, "Error compiling regex: %s\n", pcre_error(erroffset));
return 0;
}
int match_status = pcre_exec(compiled_regex, NULL, filename, strlen(filename), 0, 0, NULL, 0);
if (match_status == 0) {
// 匹配失败
fprintf(stderr, "File extension not found in %s\n", filename);
} else {
char *extension = malloc(pcre_get_substring_count(compiled_regex) + 1);
pcre_get_substring(compiled_regex, NULL, 1, extension);
printf("File extension: %s\n", extension);
free(extension);
}
pcre_free(compiled_regex);
return match_status;
}
int main() {
const char *filename = "/path/to/your/file.txt";
match_file_extension(filename, FILE_EXTENSION_REGEX);
return 0;
}
```
这个示例中,`FILE_EXTENSION_REGEX`是一个正则表达式,用于匹配文件名中的最后一个`.`字符之后的部分。请注意,这只是一个基本的示例,实际应用可能需要根据具体需求调整。
阅读全文
相关推荐
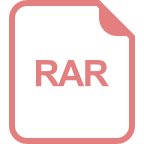
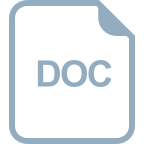
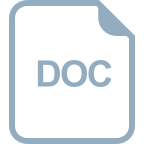
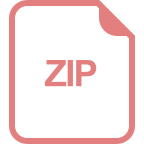
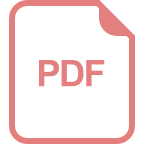
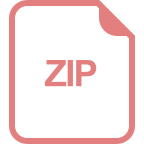
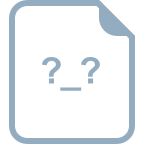
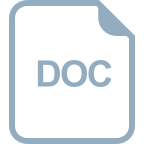
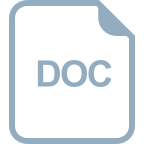
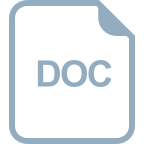

