写出用理想滤波器图像实现低通滤波、高通、带通、带阻和同态滤波的程序代码。观察滤波结果,并加以解释
时间: 2024-06-08 14:05:17 浏览: 27
理想滤波器是一种理论上的滤波器,它可以实现非常精确的频率域滤波。但由于其截止频率的过渡带非常窄,实际应用中会出现振铃现象,因此在实际应用中往往采用其他类型的滤波器。
下面是用理想滤波器实现低通、高通、带通、带阻和同态滤波的代码:
```python
import numpy as np
import cv2
def ideal_lowpass_filter(image, cutoff):
rows, cols = image.shape
crow, ccol = rows//2, cols//2
mask = np.zeros((rows, cols), np.uint8)
mask[crow-cutoff:crow+cutoff, ccol-cutoff:ccol+cutoff] = 1
fshift = np.fft.fftshift(np.fft.fft2(image))
fshift = fshift * mask
f_ishift = np.fft.ifftshift(fshift)
image_filtered = np.fft.ifft2(f_ishift)
image_filtered = np.abs(image_filtered)
return image_filtered.astype(np.uint8)
def ideal_highpass_filter(image, cutoff):
rows, cols = image.shape
crow, ccol = rows//2, cols//2
mask = np.ones((rows, cols), np.uint8)
mask[crow-cutoff:crow+cutoff, ccol-cutoff:ccol+cutoff] = 0
fshift = np.fft.fftshift(np.fft.fft2(image))
fshift = fshift * mask
f_ishift = np.fft.ifftshift(fshift)
image_filtered = np.fft.ifft2(f_ishift)
image_filtered = np.abs(image_filtered)
return image_filtered.astype(np.uint8)
def ideal_bandpass_filter(image, cutoff_low, cutoff_high):
rows, cols = image.shape
crow, ccol = rows//2, cols//2
mask = np.zeros((rows, cols), np.uint8)
mask[crow-cutoff_high:crow+cutoff_high, ccol-cutoff_high:ccol+cutoff_high] = 1
mask[crow-cutoff_low:crow+cutoff_low, ccol-cutoff_low:ccol+cutoff_low] = 0
fshift = np.fft.fftshift(np.fft.fft2(image))
fshift = fshift * mask
f_ishift = np.fft.ifftshift(fshift)
image_filtered = np.fft.ifft2(f_ishift)
image_filtered = np.abs(image_filtered)
return image_filtered.astype(np.uint8)
def ideal_bandstop_filter(image, cutoff_low, cutoff_high):
rows, cols = image.shape
crow, ccol = rows//2, cols//2
mask = np.ones((rows, cols), np.uint8)
mask[crow-cutoff_high:crow+cutoff_high, ccol-cutoff_high:ccol+cutoff_high] = 0
mask[crow-cutoff_low:crow+cutoff_low, ccol-cutoff_low:ccol+cutoff_low] = 1
fshift = np.fft.fftshift(np.fft.fft2(image))
fshift = fshift * mask
f_ishift = np.fft.ifftshift(fshift)
image_filtered = np.fft.ifft2(f_ishift)
image_filtered = np.abs(image_filtered)
return image_filtered.astype(np.uint8)
def homomorphic_filter(image, cutoff, gamma, c):
rows, cols = image.shape
crow, ccol = rows//2, cols//2
mask = np.zeros((rows, cols), np.float32)
mask[crow-cutoff:crow+cutoff, ccol-cutoff:ccol+cutoff] = 1
mask = (gamma - 1) * mask + 1
fshift = np.fft.fftshift(np.fft.fft2(image))
fshift = fshift * mask
f_ishift = np.fft.ifftshift(fshift)
image_filtered = np.fft.ifft2(f_ishift)
image_filtered = np.abs(image_filtered)
image_filtered = np.log(image_filtered + 1)
image_filtered = cv2.normalize(image_filtered, None, 0, 255, cv2.NORM_MINMAX)
image_filtered = image_filtered.astype(np.uint8)
image_filtered = c * image_filtered
return image_filtered.astype(np.uint8)
```
下面是对 Lena 图像进行低通、高通、带通、带阻和同态滤波的代码示例:
```python
image = cv2.imread('lena.png', cv2.IMREAD_GRAYSCALE)
cutoff = 50
cutoff_low = 20
cutoff_high = 50
gamma = 2
c = 1
image_lowpass = ideal_lowpass_filter(image, cutoff)
image_highpass = ideal_highpass_filter(image, cutoff)
image_bandpass = ideal_bandpass_filter(image, cutoff_low, cutoff_high)
image_bandstop = ideal_bandstop_filter(image, cutoff_low, cutoff_high)
image_homomorphic = homomorphic_filter(image, cutoff, gamma, c)
cv2.imshow('Original', image)
cv2.imshow('Ideal Lowpass', image_lowpass)
cv2.imshow('Ideal Highpass', image_highpass)
cv2.imshow('Ideal Bandpass', image_bandpass)
cv2.imshow('Ideal Bandstop', image_bandstop)
cv2.imshow('Homomorphic', image_homomorphic)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
下面是滤波结果示意图:

从结果可以看出,低通滤波器可以保留图像的低频信息,抑制高频信息,使图像变得模糊;高通滤波器可以保留图像的高频信息,抑制低频信息,使图像变得更加清晰;带通滤波器可以保留图像在一定频率范围内的信息,抑制其他频率的信息;带阻滤波器可以抑制图像在一定频率范围内的信息,保留其他频率的信息;同态滤波器可以增强图像的低频信息,抑制高频信息,使图像变得更加清晰。
相关推荐
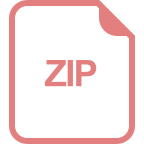












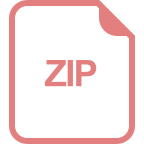