python实现Q-learning
时间: 2023-07-07 13:40:31 浏览: 96
Q-learning是一种基于值的强化学习算法,它可以学习到一个最优的策略,使得在某个环境下,智能体可以最大化累计的奖励。下面是一个简单的Python实现示例:
```python
import numpy as np
# 定义环境
env = np.array([[0, 0, 0, 0, 0],
[0, 0, -1, 0, 0],
[0, 0, 0, -1, 0],
[0, -1, 0, 1, 0],
[0, 0, 0, 0, 0]])
# 定义参数
gamma = 0.8 # 折扣因子
alpha = 0.5 # 学习率
epsilon = 0.1 # 探索率
num_episodes = 1000 # 训练轮数
# 初始化Q表
Q = np.zeros((env.shape[0], env.shape[1], 4))
# 定义动作
actions = ['up', 'down', 'left', 'right']
# Q-learning算法主循环
for episode in range(num_episodes):
# 初始化状态
state = (0, 0)
# 循环直到到达目标状态
while True:
# 选择动作
if np.random.uniform(0, 1) < epsilon:
action = np.random.choice(actions)
else:
action = actions[np.argmax(Q[state[0], state[1]])]
# 执行动作
if action == 'up':
next_state = (max(state[0]-1, 0), state[1])
elif action == 'down':
next_state = (min(state[0]+1, env.shape[0]-1), state[1])
elif action == 'left':
next_state = (state[0], max(state[1]-1, 0))
elif action == 'right':
next_state = (state[0], min(state[1]+1, env.shape[1]-1))
# 计算奖励
reward = env[next_state[0], next_state[1]]
# 更新Q表
Q[state[0], state[1], actions.index(action)] += alpha * (reward + gamma * np.max(Q[next_state[0], next_state[1]]) - Q[state[0], state[1], actions.index(action)])
# 更新状态
state = next_state
# 判断是否到达目标状态
if env[state[0], state[1]] == 1:
break
# 输出最优策略
state = (0, 0)
while True:
action = actions[np.argmax(Q[state[0], state[1]])]
print(action)
if action == 'up':
state = (max(state[0]-1, 0), state[1])
elif action == 'down':
state = (min(state[0]+1, env.shape[0]-1), state[1])
elif action == 'left':
state = (state[0], max(state[1]-1, 0))
elif action == 'right':
state = (state[0], min(state[1]+1, env.shape[1]-1))
if env[state[0], state[1]] == 1:
break
```
在这个例子中,我们使用了一个简单的5x5环境,其中0表示空格,-1表示障碍物,1表示目标状态。我们使用Q表来存储智能体对每个状态和动作的估计值,通过不断与环境交互,智能体可以学习到一个最优的Q表,从而得到最优的策略。
在主循环中,我们按照Q-learning算法的步骤循环执行,直到到达目标状态。在每个时间步,我们首先根据当前状态和Q表选择一个动作,然后执行该动作并观察下一个状态和奖励,最后更新Q表。在每个时间步中,我们使用贪心策略或epsilon-greedy策略来选择动作。
最后,我们使用已经学习到的Q表输出最优的策略。在这个例子中,我们从初始状态开始,按照Q表中估计值最大的动作依次执行,直到到达目标状态。
阅读全文
相关推荐
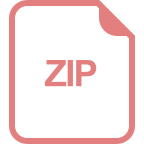
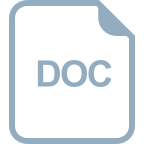


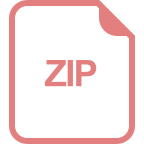
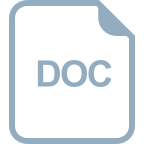
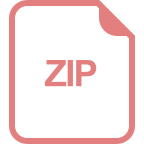
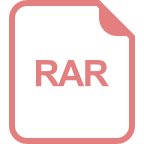
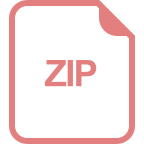
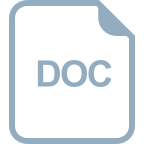
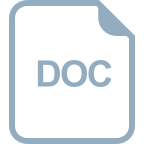

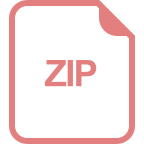
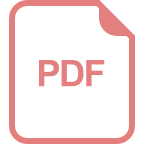
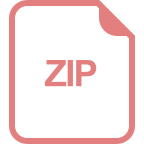

