编写类 `MyDate` 具有属性年月日,要求一个构造函数初始化属性年月日,提供重置日期、增加日期(考虑闰年闰月)、输出日期等成员函数。
时间: 2024-06-12 09:07:40 浏览: 8
以下是 `MyDate` 类的实现:
```python
class MyDate:
def __init__(self, year, month, day):
self.year = year
self.month = month
self.day = day
def reset(self, year, month, day):
self.year = year
self.month = month
self.day = day
def add_day(self, days):
days_in_month = [0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if self.is_leap_year():
days_in_month[2] = 29
while days > 0:
days_in_current_month = days_in_month[self.month]
if self.day + days > days_in_current_month:
days -= (days_in_current_month - self.day + 1)
self.day = 1
self.add_month(1)
else:
self.day += days
days = 0
def add_month(self, months):
while months > 0:
if self.month == 12:
self.year += 1
self.month = 1
else:
self.month += 1
months -= 1
def is_leap_year(self):
if self.year % 4 == 0 and (self.year % 100 != 0 or self.year % 400 == 0):
return True
else:
return False
def __str__(self):
return str(self.year) + '-' + str(self.month).zfill(2) + '-' + str(self.day).zfill(2)
```
上述代码实现了 `MyDate` 类,具有以下成员函数:
- `__init__(self, year, month, day)`:构造函数,初始化属性年月日。
- `reset(self, year, month, day)`:重置日期。
- `add_day(self, days)`:增加日期,考虑闰年闰月。
- `add_month(self, months)`:增加月份,考虑跨年。
- `is_leap_year(self)`:判断是否为闰年。
- `__str__(self)`:输出日期。
以下是一个使用示例:
```python
date = MyDate(2021, 5, 20)
print(date) # 输出 2021-05-20
date.add_day(10)
print(date) # 输出 2021-05-30
date.add_day(3)
print(date) # 输出 2021-06-02
date.add_month(10)
print(date) # 输出 2022-04-02
date.reset(2023, 12, 31)
print(date) # 输出 2023-12-31
```
相关推荐
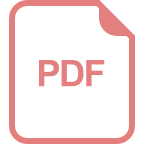
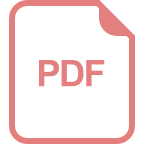
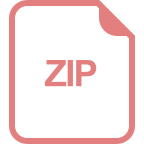














