数据结构链表实现学生成绩管理系统不及格人数并输出对应的课程号
时间: 2023-07-21 15:07:31 浏览: 49
好的,我可以给你一个基本的思路,你可以根据它自己尝试实现:
1. 定义一个结构体来存储学生信息和成绩,包括学生姓名、学号、课程号、成绩等字段。
2. 定义一个链表结构体,包括头指针和链表长度等字段。
3. 读取文件中的数据,逐行解析出学生信息和成绩,创建一个链表节点存储它们。
4. 遍历链表,统计每门课程不及格的人数,并输出对应的课程号。
具体实现的细节可以参考以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 100
// 学生信息结构体
typedef struct Student {
char name[MAX_LEN]; // 姓名
char id[MAX_LEN]; // 学号
char course[MAX_LEN]; // 课程号
int score; // 成绩
} Student;
// 链表节点结构体
typedef struct Node {
Student student; // 学生信息
struct Node* next; // 后继节点指针
} Node;
// 链表结构体
typedef struct List {
Node* head; // 头指针
int len; // 链表长度
} List;
// 创建一个链表节点
Node* create_node(Student student) {
Node* node = (Node*)malloc(sizeof(Node));
node->student = student;
node->next = NULL;
return node;
}
// 在链表尾部插入一个节点
void insert_node(List* list, Node* node) {
if (list->head == NULL) {
list->head = node;
} else {
Node* tail = list->head;
while (tail->next != NULL) {
tail = tail->next;
}
tail->next = node;
}
list->len++;
}
// 统计不及格人数
void count_failed(List* list) {
Node* p = list->head;
int count[MAX_LEN] = {0}; // 用数组记录每门课程不及格的人数
while (p != NULL) {
if (p->student.score < 60) {
count[atoi(p->student.course)]++;
}
p = p->next;
}
for (int i = 0; i < MAX_LEN; i++) {
if (count[i] > 0) {
printf("课程号:%d,不及格人数:%d\n", i, count[i]);
}
}
}
int main() {
List list = {NULL, 0};
FILE* fp = fopen("score.txt", "r");
if (fp == NULL) {
printf("文件打开失败!\n");
return 1;
}
char line[MAX_LEN];
while (fgets(line, MAX_LEN, fp) != NULL) {
Student student;
sscanf(line, "%s %s %s %d", student.name, student.id, student.course, &student.score);
Node* node = create_node(student);
insert_node(&list, node);
}
fclose(fp);
count_failed(&list);
return 0;
}
```
这个程序假设数据文件 `score.txt` 中每行依次包含学生姓名、学号、课程号、成绩,字段之间用空格隔开。它会读取文件中的数据,创建一个链表来存储学生信息和成绩,然后遍历链表,统计每门课程不及格的人数,并输出对应的课程号。
相关推荐
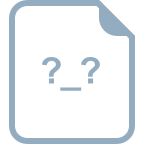
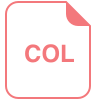
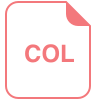
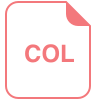
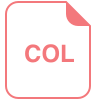
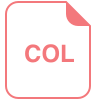









