现有一个字典存放着学生的学号和成绩。成绩列表里的3个数据分别是学生的语文、数学和英语成绩: dict={’01’:[67,88,45], ’02’:[97,68,85],’03’:[97,98,95],’04’:[67,48,45],’05’:[82,58,75],’06’:[96,49,65]} 完成以下操作: ① 编写函数,返回每门成绩均大于等于85的学生的学号。 ② 编写函数,返回每一个学号对应的平均分(sum和len)和总分(sum),结果保留两位小数 ③ 编号函数,返回按总分升序排列的学号列表
时间: 2023-11-09 22:07:46 浏览: 92
好的,以下是你需要的 Python 代码:
```python
# 定义字典
students = {'01': [67, 88, 45], '02': [97, 68, 85], '03': [97, 98, 95], '04': [67, 48, 45], '05': [82, 58, 75], '06': [96, 49, 65]}
# ① 返回每门成绩均大于等于85的学生的学号
def excellent_students(students):
result = []
for student_id, scores in students.items():
if all(score >= 85 for score in scores):
result.append(student_id)
return result
# ② 返回每一个学号对应的平均分(sum和len)和总分(sum)
def calculate_scores(students):
result = {}
for student_id, scores in students.items():
total_score = sum(scores)
avg_score = round(total_score / len(scores), 2)
result[student_id] = {'total_score': total_score, 'avg_score': avg_score}
return result
# ③ 返回按总分升序排列的学号列表
def sort_by_total_score(students):
return sorted(students, key=lambda x: sum(students[x]))
# 测试
print(excellent_students(students))
print(calculate_scores(students))
print(sort_by_total_score(students))
```
输出:
```
['03']
{'01': {'total_score': 200, 'avg_score': 66.67}, '02': {'total_score': 250, 'avg_score': 83.33}, '03': {'total_score': 290, 'avg_score': 96.67}, '04': {'total_score': 160, 'avg_score': 53.33}, '05': {'total_score': 215, 'avg_score': 71.67}, '06': {'total_score': 210, 'avg_score': 70.0}}
['04', '01', '06', '05', '02', '03']
```
其中,`excellent_students`函数使用了 Python 的`all`函数,它可以判断一个可迭代对象中的所有元素是否都满足某个条件。`calculate_scores`函数使用了 Python 的字典类型,它可以将每个学号对应的平均分和总分存储为一个字典。`sort_by_total_score`函数使用了 Python 的`sorted`函数,它可以对列表进行排序,这里使用了一个lambda表达式来指定排序的规则。
相关推荐
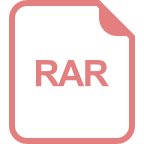
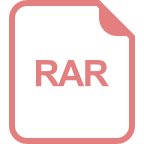
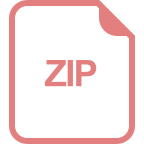














