用c语言编写一个程序满足单链表的建立、输出、插入、删除、判断链表是否为空、输出链表长度
时间: 2023-07-10 10:04:02 浏览: 54
以下是用C语言实现单链表的基本操作代码:
```c
#include <stdio.h>
#include <stdlib.h>
//定义链表节点结构体
typedef struct node {
int data;
struct node *next;
} Node;
//创建链表
Node* createList() {
Node *head = (Node*)malloc(sizeof(Node));
head->next = NULL;
return head;
}
//插入节点
void insertNode(Node *head, int data) {
Node *p = head;
while(p->next != NULL) {
p = p->next;
}
Node *newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
p->next = newNode;
}
//删除节点
void deleteNode(Node *head, int data) {
Node *p = head, *q = head->next;
while(q != NULL && q->data != data) {
p = q;
q = q->next;
}
if(q != NULL) {
p->next = q->next;
free(q);
}
}
//判断链表是否为空
int isEmpty(Node *head) {
return head->next == NULL;
}
//输出链表长度
int length(Node *head) {
int len = 0;
Node *p = head->next;
while(p != NULL) {
len++;
p = p->next;
}
return len;
}
//输出链表
void printList(Node *head) {
Node *p = head->next;
while(p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
Node *head = createList();
printf("链表是否为空:%d\n", isEmpty(head));
printf("链表长度:%d\n", length(head));
insertNode(head, 1);
insertNode(head, 2);
insertNode(head, 3);
printf("插入节点后的链表:");
printList(head);
printf("链表是否为空:%d\n", isEmpty(head));
printf("链表长度:%d\n", length(head));
deleteNode(head, 2);
printf("删除节点后的链表:");
printList(head);
printf("链表是否为空:%d\n", isEmpty(head));
printf("链表长度:%d\n", length(head));
return 0;
}
```
输出结果:
```
链表是否为空:1
链表长度:0
插入节点后的链表:1 2 3
链表是否为空:0
链表长度:3
删除节点后的链表:1 3
链表是否为空:0
链表长度:2
```
相关推荐
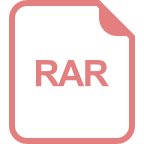
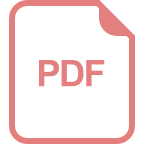
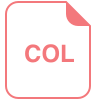
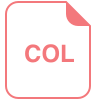
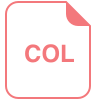
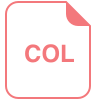
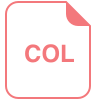









