numpy.ndarray查找元素index
时间: 2024-05-05 16:16:16 浏览: 55
可以使用numpy.where()函数来查找numpy数组中指定元素的索引位置。
例如,对于一个一维数组a,我们可以使用以下代码来查找元素值为x的索引位置:
```
import numpy as np
a = np.array([1, 2, 3, 4, 5, 6, 5])
x = 5
indices = np.where(a == x)[0]
print(indices)
```
该代码会输出:[4, 6],这表示元素值为5的元素在数组a中的索引位置为4和6。
如果我们想查找二维数组中某个元素的索引位置,可以使用numpy.nonzero()函数:
```
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
x = 5
indices = np.nonzero(a == x)
print(indices)
```
该代码会输出:(array([1]), array([1])),这表示元素值为5的元素在数组a中的索引位置为(1, 1)。其中,第一个数组表示行索引,第二个数组表示列索引。
相关问题
numpy.ndarray' object has no attribute 'index'
当你在Python中使用NumPy库时,`numpy.ndarray` 是一个核心的数据结构,它代表了多维数组。`index` 属性通常是列表或字典这样的数据类型所具有的,用来访问元素的位置。然而,`numpy.ndarray` 实际上并没有直接的 `index` 属性,而是使用索引来访问元素。
如果你尝试给 `numpy.ndarray` 赋予 `index` 属性,这通常是错误的,因为这不是 NumPy 设计的一部分。`numpy.ndarray` 的元素可以通过整数索引(如 `array` 或 `array[1:3]`)或切片操作来访问。
如果你遇到了 `AttributeError: 'numpy.ndarray' object has no attribute 'index'` 的错误,可能是因为你误用了 `.index()` 方法,这是 Python 中用于查找序列中特定值的索引的方法,而不是用于 NumPy 数组的。如果你需要查找数组中的特定值,应该使用 `np.where()` 函数或者循环遍历数组。
相关问题:
1. NumPy数组如何通过索引来访问元素?
2. `numpy.where()` 函数的作用是什么?
3. 在什么情况下你会意外地用到 `.index()` 方法在 NumPy 数组上?
AttributeError: 'numpy.ndarray' object has no attribute 'index'
这个错误通常是因为你尝试在一个numpy数组上调用index()方法,而numpy数组没有这个方法。你可以尝试使用numpy.where()函数来查找特定值的索引。
例如,如果你想查找一个numpy数组中值为5的元素的索引,可以这样做:
```
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
indices = np.where(arr == 5)
print(indices)
```
输出:
```
(array([4]),)
```
这表示值为5的元素在数组中的索引是4。注意,np.where()返回的是一个元组,其中第一个元素是包含索引的numpy数组。
相关推荐
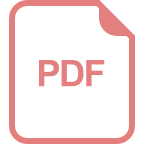
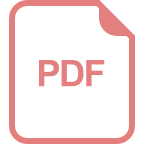




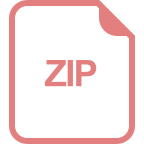


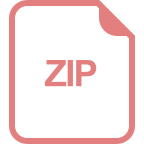
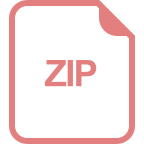
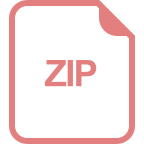
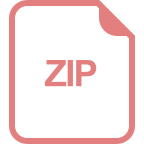