print(row_ind.index(i)) AttributeError: 'numpy.ndarray' object has no attribute 'index'
时间: 2024-05-30 17:06:51 浏览: 153
这个错误是因为numpy中的ndarray对象没有index方法,所以无法使用该方法。如果你想要获取某个元素在ndarray中的索引,可以使用numpy中的where方法。例如,可以使用以下代码来获取i在ndarray row_ind中的索引:
```
import numpy as np
row_ind = np.array([1, 2, 3, 4])
indexes = np.where(row_ind == i)
print(indexes)
```
其中i为要查找的元素。np.where会返回一个tuple,其中第一个元素是包含查找元素索引的ndarray,因此我们需要使用indexes来获取索引。
相关问题
In file included from /home/yhdr/2-test-2023-06_v3/sent.h:24:0, from /home/yhdr/2-test-2023-06_v3/sent.cpp:1: /usr/include/c++/7/thread: In instantiation of ‘struct std::thread::_Invoker<std::tuple<void (*)(double*, double&, double&, double&, double&, double&), double**, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double> > >’: /usr/include/c++/7/thread:127:22: required from ‘std::thread::thread(_Callable&&, _Args&& ...) [with _Callable = void (&)(double*, double&, double&, double&, double&, double&); _Args = {double**, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double>}]’ /home/yhdr/2-test-2023-06_v3/sent.cpp:18:153: required from here /usr/include/c++/7/thread:240:2: error: no matching function for call to ‘std::thread::_Invoker<std::tuple<void (*)(double*, double&, double&, double&, double&, double&), double**, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double> > >::_M_invoke(std::thread::_Invoker<std::tuple<void (*)(double*, double&, double&, double&, double&, double&), double**, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double> > >::_Indices)’ operator()() ^~~~~~~~ /usr/include/c++/7/thread:231:4: note: candidate: template<long unsigned int ..._Ind> decltype (std::__invoke((_S_declval<_Ind>)()...)) std::thread::_Invoker<_Tuple>::_M_invoke(std::_Index_tuple<_Ind ...>) [with long unsigned int ..._Ind = {_Ind ...}; _Tuple = std::tuple<void (*)(double*, double&, double&, double&, double&, double&), double**, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double>, std::reference_wrapper<double> >] _M_invoke(_Index_tuple<_Ind...>)
这个编译错误看起来是在创建一个std::thread对象时出现了问题。它指出无法匹配std::thread::_Invoker模板的_M_invoke函数的参数列表。这通常是因为您的函数参数与std::thread的参数不匹配,或者您的函数参数中有无法转换的类型。
请检查您的函数参数类型是否与std::thread需要的参数类型相匹配。如果您的函数需要接受其他参数类型,请尝试将它们转换为std::reference_wrapper或其他可转换的类型。您可能还需要检查您的函数参数数量是否正确。
如果您需要更多的帮助,请提供更多的代码和上下文,我可以更好地帮助您解决这个问题。
from scipy.stats import ttest_ind for i in range(0,len(otu_map_match.index)): if i+1>len(otu_map_match.index): break pvalue = pd.DataFrame() tvalue = pd.DataFrame() tmp1 = otu_map_match.filter(like=otu_map_match.index[i],axis=0).drop(['#SampleID','Group'],axis=1) tmp2 = otu_map_match.filter(like=otu_map_match.index[i+1],axis=0).drop(['#SampleID','Group'],axis=1) for j in range(0,len(otu_map_match.columns)): pvalue.loc[tmp1.index[i],tmp2.index[i]] = ttest_ind(tmp1.iloc[:,j],tmp2.iloc[:,j])[1] tvalue.loc[tmp1.index[i],tmp2.index[i]] = ttest_ind(tmp1.iloc[:,j],tmp2.iloc[:,j])[0]
这段代码没有明显的语法错误,但是其中有一些潜在的逻辑问题:
1. 在判断i+1是否大于len(otu_map_match.index)时,应该使用小于号而不是大于号,否则会跳过最后一个样本;
2. 在for循环中,pvalue和tvalue的行索引和列索引都是用的i而不是j,这样会导致结果被覆盖,应该将i改为j;
3. 在使用ttest_ind函数时,需要确保样本之间的数据长度相等,否则会报错,这个问题需要根据具体数据进行处理。
下面是修改后的代码,您可以参考一下:
```python
from scipy.stats import ttest_ind
pvalue = pd.DataFrame()
tvalue = pd.DataFrame()
for i in range(len(otu_map_match.index) - 1):
tmp1 = otu_map_match.filter(like=otu_map_match.index[i], axis=0).drop(['#SampleID','Group'], axis=1)
tmp2 = otu_map_match.filter(like=otu_map_match.index[i+1], axis=0).drop(['#SampleID','Group'], axis=1)
for j in range(len(otu_map_match.columns)):
if len(tmp1.iloc[:,j]) != len(tmp2.iloc[:,j]):
continue
pvalue.loc[tmp1.columns[j], tmp2.columns[j]] = ttest_ind(tmp1.iloc[:,j], tmp2.iloc[:,j])[1]
tvalue.loc[tmp1.columns[j], tmp2.columns[j]] = ttest_ind(tmp1.iloc[:,j], tmp2.iloc[:,j])[0]
```
希望能对您有所帮助。
阅读全文
相关推荐
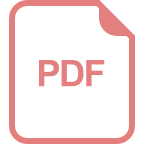
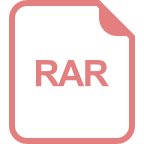















