用qt写一个电路网孔自动圈划算法不用界面,将网孔节点写入SQLite 数据库
时间: 2023-05-23 11:03:18 浏览: 43
可以使用以下步骤实现电路网孔自动圈划算法:
1. 从SQLite数据库中读取网孔节点信息,包括节点坐标和连接关系。
2. 使用一个算法生成所有可能的圈内网孔组合。比如,可以使用 DFS 等算法遍历网络图。
3. 对于每个圈内网孔组合,使用一个凸包算法计算出该组合的凸包多边形。
4. 对比不同圈内网孔组合的凸包面积,并选择面积最小的一个作为最终圈选结果。
5. 将最终圈选结果存储到SQLite数据库中,以便后续使用。
示例代码:
```cpp
#include <QDebug>
#include <QSqlDatabase>
#include <QSqlQuery>
#include <QSqlError>
#include <QtMath>
struct Point {
qreal x, y;
Point(qreal x, qreal y) {this->x = x; this->y = y;}
};
QVector<Point> getNodesFromDB() {
QVector<Point> nodes;
QSqlDatabase db = QSqlDatabase::database();
QSqlQuery query(db);
query.prepare("SELECT id, x, y FROM nodes");
if (query.exec()) {
while (query.next()) {
qreal x = query.value(1).toDouble();
qreal y = query.value(2).toDouble();
Point node(x, y);
nodes.append(node);
}
} else {
qDebug() << "Database error: " << query.lastError().text();
}
return nodes;
}
QVector<QVector<Point>> findCombinations(const QVector<Point>& nodes) {
QVector<QVector<Point>> combinations;
// TODO: 使用 DFS 等算法生成圈内网孔组合
return combinations;
}
qreal area(const QVector<Point>& polygon) {
if (polygon.size() < 3) return 0;
qreal sum = 0;
for (int i = 0; i < polygon.size() - 1; i++) {
sum += polygon[i].x * polygon[i + 1].y - polygon[i].y * polygon[i + 1].x;
}
sum += polygon.last().x * polygon.first().y - polygon.last().y * polygon.first().x;
return qAbs(sum) / 2;
}
QVector<Point> convexHull(QVector<Point>& points) {
qSort(points.begin(), points.end(), [](const Point& a, const Point& b){
if (a.x == b.x) return a.y < b.y;
return a.x < b.x;
});
QVector<Point> upperHull, lowerHull;
for (int i = 0; i < points.size(); i++) {
while (upperHull.size() > 1 && (points[i].y - upperHull.last().y) * (upperHull[upperHull.size() - 2].x - upperHull.last().x) >= (points[i].x - upperHull.last().x) * (upperHull[upperHull.size() - 2].y - upperHull.last().y)) {
upperHull.pop_back();
}
upperHull.push_back(points[i]);
}
lowerHull.push_back(points.last());
for (int i = points.size() - 2; i >= 0; i--) {
while (lowerHull.size() > 1 && (points[i].y - lowerHull.last().y) * (lowerHull[lowerHull.size() - 2].x - lowerHull.last().x) >= (points[i].x - lowerHull.last().x) * (lowerHull[lowerHull.size() - 2].y - lowerHull.last().y)) {
lowerHull.pop_back();
}
lowerHull.push_back(points[i]);
}
lowerHull.pop_front();
lowerHull.pop_back();
QVector<Point> result = upperHull + lowerHull;
return result;
}
QVector<Point> getSmallestConvexHull(const QVector<QVector<Point>>& possibleHulls) {
QVector<Point> smallestHull;
qreal smallestArea = 1e20;
for (const auto& hull : possibleHulls) {
qreal hullArea = area(hull);
if (hullArea < smallestArea) {
smallestArea = hullArea;
smallestHull = hull;
}
}
return smallestHull;
}
void storeResultIntoDB(const QVector<Point>& points) {
QSqlDatabase db = QSqlDatabase::database();
QSqlQuery query(db);
query.prepare("INSERT INTO selections (point_count, polygon) VALUES (:point_count, :polygon)");
query.bindValue(":point_count", points.size());
QStringList pointStrs;
for (const auto& point : points) {
pointStrs.append(QString::number(point.x) + "," + QString::number(point.y));
}
QString polygonStr = pointStrs.join(";");
query.bindValue(":polygon", polygonStr);
if (!query.exec()) {
qDebug() << "Database error: " << query.lastError().text();
}
}
void automaticCircuitSelection() {
QVector<Point> nodes = getNodesFromDB();
QVector<QVector<Point>> possibleHulls = findCombinations(nodes);
QVector<Point> smallestHull = getSmallestConvexHull(possibleHulls);
storeResultIntoDB(smallestHull);
}
int main(int argc, char *argv[]) {
QSqlDatabase db = QSqlDatabase::addDatabase("QSQLITE");
db.setDatabaseName(":memory:");
if (!db.open()) {
qDebug() << "Database error: " << db.lastError().text();
return 1;
}
QSqlQuery query(db);
query.exec("CREATE TABLE nodes (id INTEGER PRIMARY KEY AUTOINCREMENT, x REAL, y REAL)");
query.exec("CREATE TABLE selections (id INTEGER PRIMARY KEY AUTOINCREMENT, point_count INTEGER, polygon TEXT)");
query.prepare("INSERT INTO nodes (x, y) VALUES (:x, :y)");
for (int i = 0; i < 10; i++) {
query.bindValue(":x", qrand() % 100);
query.bindValue(":y", qrand() % 100);
if (!query.exec()) {
qDebug() << "Database error: " << query.lastError().text();
}
}
automaticCircuitSelection();
return 0;
}
```
这段代码实现了一个简单的电路网孔自动圈划算法,并将结果存储到 SQLite 数据库中。
相关推荐
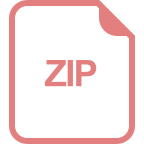
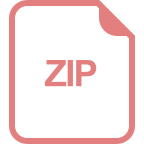
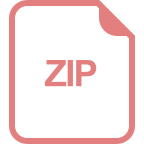














