void* malloc(size_t size)用C++怎么写
时间: 2024-05-16 10:16:57 浏览: 23
在C++中,可以使用`new`和`delete`操作符来代替`malloc`和`free`函数。`new`操作符用于动态分配内存,`delete`操作符用于释放动态分配的内存。
例如,使用`new`操作符分配内存:
```c++
int* ptr = new int[10];
```
使用`delete`操作符释放内存:
```c++
delete[] ptr;
```
注意,使用`new`操作符分配的内存必须使用`delete`操作符释放,而不能使用`free`函数。反之,使用`malloc`函数分配的内存必须使用`free`函数释放,而不能使用`delete`操作符。
相关问题
15 33 C:\Users\hp\Desktop\计?慊?\c++\c++?废?\11.14.cpp [Error] too many arguments to function 'void* malloc(size_t)'
您在使用C++编写代码时,可能会遇到“too many arguments to function 'void* malloc(size_t)'”这个错误。这个错误通常是由于在调用malloc函数时传递了太多的参数而导致的。malloc函数只需要一个参数,即要分配的内存块的大小,因此如果您传递了多个参数,则会出现此错误。
解决此问题的方法是检查您的代码并确保在调用malloc函数时只传递一个参数,即要分配的内存块的大小。如果您需要分配多个内存块,则应该多次调用malloc函数,每次只分配一个内存块。
以下是一个示例代码,演示了如何使用malloc函数分配内存块:
```c++
#include <stdlib.h>
int main() {
int *ptr;
int n = 5;
// 分配内存块
ptr = (int*) malloc(n * sizeof(int));
// 检查内存是否成功分配
if (ptr == NULL) {
printf("Memory allocation failed\n");
exit(1);
}
// 使用内存块
for (int i = 0; i < n; i++) {
ptr[i] = i + 1;
}
// 释放内存块
free(ptr);
return 0;
}
```
pullflowmonitor.cpp: In member function 'bool PullFlowMonitor::initModel()': pullflowmonitor.cpp:1223:99: error: cannot convert 'uint8_t* {aka unsigned char*}' to 'void**' for argument '1' to 'aclError aclrtMallocCached(void**, size_t, aclrtMemMallocPolicy)' AclInputs.inputs[i].data,sizeof(uint8_t)*inputsize,ACL_MEM_MALLOC_HUGE_FIRST);
这个错误是因为aclrtMallocCached函数的第一个参数是一个指向void*类型的指针,而你的代码中传递的是一个指向uint8_t*类型的指针,这样会导致类型不匹配,编译器无法编译通过。
解决这个问题,可以将aclrtMallocCached函数的第一个参数的类型改为void*类型的指针。
例如:
```c++
void* buffer;
aclError ret = aclrtMallocCached(&buffer, size, ACL_MEM_MALLOC_HUGE_FIRST);
```
或者使用reinterpret_cast进行类型转换:
```c++
uint8_t* buffer;
aclError ret = aclrtMallocCached(reinterpret_cast<void**>(&buffer), size, ACL_MEM_MALLOC_HUGE_FIRST);
```
这样就可以避免这个编译错误了。
相关推荐
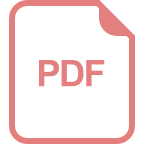
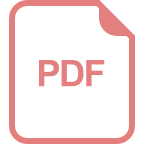












