感知器与ADALINE 的区别
时间: 2024-04-02 07:36:01 浏览: 19
感知器和ADALINE都是二元分类模型,但是它们有一些区别:
1. 感知器是一种简单的线性分类器,只能处理线性可分问题,而ADALINE是一种连续的线性分类器,可以处理非线性可分问题。
2. 感知器的输出是一个二元值(+1或-1),而ADALINE的输出是一个连续的实数值,可以表示不同类别之间的概率差异。
3. 感知器使用的是阶跃函数作为激活函数,而ADALINE使用的是线性函数或者sigmoid函数作为激活函数。
4. 感知器使用的是随机梯度下降(SGD)算法进行权重更新,而ADALINE使用的是最小均方(MSE)误差函数进行权重更新。
总的来说,ADALINE是感知器的一种改进,它能够处理更加复杂的分类问题,并且输出更加准确的结果。
相关问题
比较一下感知器和ADALINE
感知器和ADALINE都是神经网络的经典模型。
感知器是一种二元分类器,其基本原理是将输入的特征向量加权求和并加上一个偏置项,然后将结果传递给一个激活函数,最终输出二元分类结果。感知器模型的学习算法是通过不断调整权重和偏置项来最小化误差的。
ADALINE(自适应线性元件)是感知器的一种改进型,主要区别在于输出结果不再是二元分类,而是一个连续值,可以用来进行回归分析。ADALINE的学习算法则是通过最小化均方误差来调整权重和偏置项。
因此,感知器和ADALINE在原理上有一些相似之处,但是ADALINE的输出更加灵活,可以处理连续值输出,而感知器只能输出二元分类结果。
ADALINE和逻辑回归的区别
ADALINE(自适应线性神经元)和逻辑回归是两种不同的分类算法。
ADALINE是一种单层神经网络,它使用线性激活函数,并通过最小化误差平方和来学习权重。ADALINE的输出是一个连续值,需要将其转换为二进制输出进行分类。
逻辑回归是一种广泛使用的分类算法,它使用逻辑函数(如Sigmoid函数)作为激活函数,并通过最大似然估计来学习权重。逻辑回归的输出是二进制值,直接用于分类。
因此,ADALINE和逻辑回归的主要区别在于它们的激活函数和训练方法。ADALINE在处理线性可分问题时表现良好,但对于非线性问题的处理能力较差。而逻辑回归可以处理非线性问题,并且在分类问题中表现出色。
相关推荐
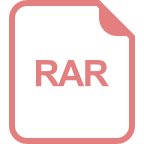
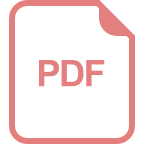











