使用visual studio2019写C语言代码,用后缀法完成四则运算表达式求解(要求手动输入原始表达式后输出后缀表达式和计算结果)。
时间: 2024-04-01 21:31:40 浏览: 125
好的,这是一个比较完整的代码,包括了输入、转换、计算和输出的过程。可以直接在Visual Studio 2019中新建一个C语言控制台应用程序,将以下代码复制到main函数中即可。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAXSIZE 100 // 定义栈的最大容量
// 定义运算符优先级
int priority(char op) {
switch (op) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
// 中缀表达式转换为后缀表达式
int infix2postfix(char *infix, char *postfix) {
char stack[MAXSIZE]; // 定义栈
int top = -1; // 栈顶指针
int i = 0, j = 0; // i遍历输入的中缀表达式,j指向输出的后缀表达式
while (infix[i] != '\0') {
if (isdigit(infix[i])) {
postfix[j++] = infix[i++];
while (isdigit(infix[i])) { // 处理多位数字
postfix[j++] = infix[i++];
}
postfix[j++] = ' '; // 数字后面加个空格
}
else if (infix[i] == '(') {
stack[++top] = infix[i++];
}
else if (infix[i] == ')') {
while (stack[top] != '(') {
postfix[j++] = stack[top--];
postfix[j++] = ' ';
}
top--; // 弹出左括号
i++;
}
else if (infix[i] == '+' || infix[i] == '-' || infix[i] == '*' || infix[i] == '/') {
while (top >= 0 && priority(stack[top]) >= priority(infix[i])) {
postfix[j++] = stack[top--];
postfix[j++] = ' ';
}
stack[++top] = infix[i++];
}
else { // 忽略空格等非法字符
i++;
}
}
while (top >= 0) { // 将栈中剩余的运算符弹出
postfix[j++] = stack[top--];
postfix[j++] = ' ';
}
postfix[j] = '\0'; // 后缀表达式字符串以'\0'结尾
return 0;
}
// 计算后缀表达式
int calc_postfix(char *postfix, double *result) {
double stack[MAXSIZE]; // 定义栈
int top = -1; // 栈顶指针
int i = 0;
double x, y, z;
while (postfix[i] != '\0') {
if (isdigit(postfix[i])) {
sscanf(postfix + i, "%lf", &z); // 从字符串中读取数字
stack[++top] = z; // 数字入栈
while (isdigit(postfix[i]) || postfix[i] == '.') { // 跳过数字
i++;
}
i++; // 跳过空格
}
else if (postfix[i] == '+' || postfix[i] == '-' || postfix[i] == '*' || postfix[i] == '/') {
y = stack[top--]; // 弹出栈顶元素
x = stack[top--];
switch (postfix[i++]) {
case '+':
z = x + y;
break;
case '-':
z = x - y;
break;
case '*':
z = x * y;
break;
case '/':
z = x / y;
break;
}
stack[++top] = z; // 运算结果入栈
}
else { // 忽略空格等非法字符
i++;
}
}
*result = stack[top]; // 最终结果为栈中唯一元素
return 0;
}
int main() {
char infix[MAXSIZE], postfix[MAXSIZE];
double result;
printf("请输入中缀表达式:\n");
fgets(infix, MAXSIZE, stdin); // 从标准输入中读取一行字符
infix2postfix(infix, postfix);
printf("后缀表达式为:%s\n", postfix);
calc_postfix(postfix, &result);
printf("计算结果为:%f\n", result);
return 0;
}
```
这个程序可以处理整数和小数的四则运算表达式,支持加、减、乘、除、括号等运算符,可以自动跳过空格等非法字符。使用时只需要在控制台中输入中缀表达式,程序会自动输出后缀表达式和计算结果。
阅读全文
相关推荐
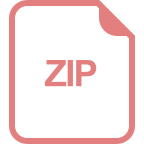
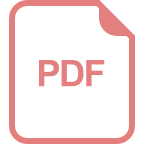
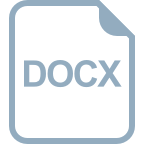
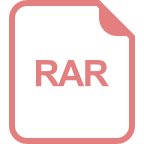
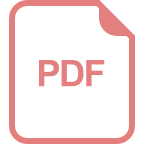
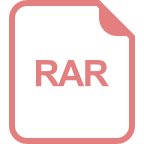
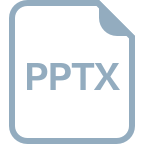
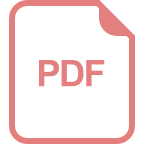
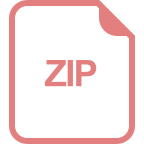