定义pso+xgboost多分类python
时间: 2023-09-10 20:09:14 浏览: 201
PSO (Particle Swarm Optimization)是一种优化算法,而XGBoost是基于GBDT(梯度提升决策树)的机器学习算法。它们可以结合在一起用于多分类问题的解决。
下面是一个使用PSO和XGBoost进行多分类的Python示例代码:
首先,导入必要的库:
```python
import numpy as np
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from xgboost import XGBClassifier
from pyswarms.discrete import BinaryPSO
```
接着,生成一个人工数据集:
```python
X, y = make_classification(n_samples=1000, n_classes=4, n_features=10, n_clusters_per_class=1, random_state=42)
```
将数据集划分为训练集和测试集:
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
定义一个评价函数,用于计算模型的精度:
```python
def accuracy(y_true, y_pred):
return np.sum(y_true == y_pred) / len(y_true)
```
定义PSO的适应度函数,即将二进制向量转换为XGBoost的超参数:
```python
def pso_fit_function(x, X_train, y_train, X_test, y_test):
n_estimators = int(x[0])
max_depth = int(x[1])
learning_rate = x[2]
gamma = x[3]
subsample = x[4]
colsample_bytree = x[5]
model = XGBClassifier(n_estimators=n_estimators, max_depth=max_depth, learning_rate=learning_rate, gamma=gamma, subsample=subsample, colsample_bytree=colsample_bytree, random_state=42)
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
return -accuracy(y_test, y_pred)
```
定义PSO的超参数和范围:
```python
pso_options = {'c1': 0.5, 'c2': 0.3, 'w': 0.9}
pso_bounds = (np.array([50, 1, 0.001, 0, 0.1, 0.1]), np.array([200, 10, 1, 5, 1, 1]))
```
运行PSO算法:
```python
pso_optimizer = BinaryPSO(n_particles=10, dimensions=6, options=pso_options, bounds=pso_bounds)
pso_cost, pso_pos = pso_optimizer.optimize(pso_fit_function, iters=10, X_train=X_train, y_train=y_train, X_test=X_test, y_test=y_test)
```
最后,使用PSO得到的超参数训练XGBoost模型,并对测试集进行预测:
```python
n_estimators = int(pso_pos[0])
max_depth = int(pso_pos[1])
learning_rate = pso_pos[2]
gamma = pso_pos[3]
subsample = pso_pos[4]
colsample_bytree = pso_pos[5]
model = XGBClassifier(n_estimators=n_estimators, max_depth=max_depth, learning_rate=learning_rate, gamma=gamma, subsample=subsample, colsample_bytree=colsample_bytree, random_state=42)
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
print('Accuracy:', accuracy(y_test, y_pred))
```
这就是使用PSO和XGBoost进行多分类的Python代码。
阅读全文
相关推荐
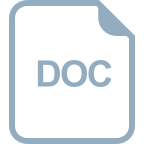
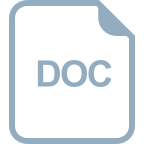
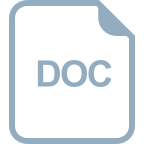











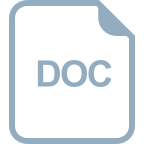
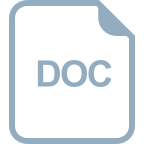
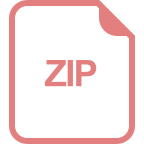