pso-xgboost多分类python
时间: 2023-07-24 19:26:31 浏览: 224
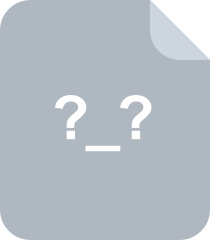
XGboost做分类
PSO-XGBoost是一种使用粒子群优化(PSO)算法来优化XGBoost模型参数的方法,用于解决多分类问题。
以下是一个使用Python实现PSO-XGBoost多分类的示例代码:
```
# 导入必要的库
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
import xgboost as xgb
from pyswarm import pso
# 加载数据集
data = pd.read_csv('data.csv')
# 将类别标签转换为数值
data['label'] = pd.factorize(data['label'])[0]
# 分割数据集
X_train, X_test, y_train, y_test = train_test_split(data.drop('label', axis=1), data['label'], test_size=0.2)
# 定义XGBoost模型函数
def xgboost_model(params, X_train, y_train, X_test, y_test):
# 提取参数
max_depth = int(params[0])
learning_rate = params[1]
n_estimators = int(params[2])
gamma = params[3]
subsample = params[4]
colsample_bytree = params[5]
# 构建XGBoost模型
model = xgb.XGBClassifier(max_depth=max_depth, learning_rate=learning_rate, n_estimators=n_estimators, gamma=gamma, subsample=subsample, colsample_bytree=colsample_bytree)
# 训练模型
model.fit(X_train, y_train)
# 预测测试集
y_pred = model.predict(X_test)
# 计算准确率
accuracy = accuracy_score(y_test, y_pred)
# 返回准确率
return 1 - accuracy
# 定义参数空间
lb = [3, 0.01, 50, 0, 0.1, 0.1]
ub = [10, 0.3, 500, 5, 1, 1]
bounds = (lb, ub)
# 运行PSO算法
xopt, fopt = pso(xgboost_model, lb, ub, args=(X_train, y_train, X_test, y_test), swarmsize=100, maxiter=50)
# 提取最优参数
max_depth = int(xopt[0])
learning_rate = xopt[1]
n_estimators = int(xopt[2])
gamma = xopt[3]
subsample = xopt[4]
colsample_bytree = xopt[5]
# 构建最优XGBoost模型
model = xgb.XGBClassifier(max_depth=max_depth, learning_rate=learning_rate, n_estimators=n_estimators, gamma=gamma, subsample=subsample, colsample_bytree=colsample_bytree)
# 训练模型
model.fit(X_train, y_train)
# 预测测试集
y_pred = model.predict(X_test)
# 计算准确率
accuracy = accuracy_score(y_test, y_pred)
# 打印准确率
print('Accuracy:', accuracy)
```
在该示例中,首先将类别标签转换为数值,然后将数据集分割为训练集和测试集。接下来,定义了一个XGBoost模型函数,该函数接受一组参数并返回模型的准确率。然后定义了一个参数空间,该空间定义了每个参数的范围。最后,使用PSO算法搜索参数空间中的最优解,并构建最终的XGBoost模型。
需要注意的是,PSO-XGBoost的参数优化结果可能会因数据集和任务的不同而不同。因此,建议在使用该方法时进行实验,并对结果进行评估。
阅读全文
相关推荐






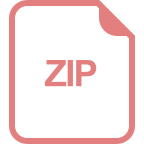
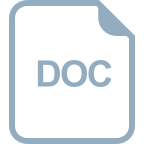




