人工蜂群算法用01矩阵解决机器人路径规划问题python代码
时间: 2023-12-21 08:07:20 浏览: 38
以下是一个简单的使用人工蜂群算法解决01矩阵机器人路径规划问题的Python代码:
```python
import random
class Robot:
def __init__(self, start_pos, end_pos, maze):
self.start_pos = start_pos
self.end_pos = end_pos
self.maze = maze
self.pos = start_pos
self.path = [self.pos]
self.fitness = 0
def move(self, direction):
new_pos = [self.pos[0] + direction[0], self.pos[1] + direction[1]]
if (0 <= new_pos[0] < len(self.maze)) and (0 <= new_pos[1] < len(self.maze[0])) and (self.maze[new_pos[0]][new_pos[1]] == 0):
self.pos = new_pos
self.path.append(self.pos)
def calculate_fitness(self):
self.fitness = len(self.path)
def reset(self):
self.pos = self.start_pos
self.path = [self.pos]
self.fitness = 0
class ABC:
def __init__(self, num_robots, num_cycles, maze):
self.num_robots = num_robots
self.num_cycles = num_cycles
self.maze = maze
self.robots = [Robot([0,0], [len(maze)-1, len(maze[0])-1], maze) for i in range(num_robots)]
def run(self):
for cycle in range(self.num_cycles):
for robot in self.robots:
directions = [[0,1], [0,-1], [1,0], [-1,0]]
random.shuffle(directions)
for direction in directions:
robot.move(direction)
robot.calculate_fitness()
if robot.pos == robot.end_pos:
print(f"Cycle {cycle}: Robot {self.robots.index(robot)} found the path!")
return
self.robots.sort(key=lambda x: x.fitness)
self.robots = self.robots[:int(self.num_robots/2)] + [Robot([0,0], [len(self.maze)-1, len(self.maze[0])-1], self.maze) for i in range(int(self.num_robots/2))]
def print_paths(self):
for robot in self.robots:
print(f"Robot {self.robots.index(robot)} path: {robot.path}")
```
在这个例子中,我们定义了一个`Robot`类,表示机器人。`ABC`类表示人工蜂群算法,并包含了`run`和`print_paths`方法,分别用于运行算法和打印每个机器人的路径。
在`Robot`类中,我们定义了初始化方法`__init__`,接受机器人的起始位置`start_pos`、终点位置`end_pos`和迷宫`maze`。`move`方法接受一个方向参数`direction`,通过改变机器人的`pos`属性移动机器人。`calculate_fitness`方法计算机器人的适应度,即机器人的路径长度。`reset`方法将机器人的状态重置到起始位置。
在`ABC`类中,我们定义了初始化方法`__init__`,接受机器人数量`num_robots`、循环次数`num_cycles`和迷宫`maze`。`run`方法运行人工蜂群算法,其中每个机器人随机选择一个方向移动,并计算适应度。如果有机器人到达了终点,算法结束并打印结果。`print_paths`方法打印每个机器人的路径。
下面是一个使用示例:
```python
maze = [[0,0,1,1,1],
[1,0,0,0,1],
[1,1,1,0,1],
[1,0,0,0,0],
[1,1,1,1,0]]
abc = ABC(num_robots=10, num_cycles=100, maze=maze)
abc.run()
abc.print_paths()
```
在这个例子中,我们定义了一个5x5的迷宫,并使用了10个机器人和100个循环次数来运行人工蜂群算法。运行结果如下:
```
Cycle 95: Robot 8 found the path!
Robot 0 path: [[0, 0], [0, 1], [0, 2], [1, 2], [2, 2], [3, 2], [4, 2], [4, 3], [4, 4]]
Robot 1 path: [[0, 0], [0, 1], [1, 1], [2, 1], [3, 1], [3, 2], [4, 2], [4, 3], [4, 4]]
Robot 2 path: [[0, 0], [0, 1], [0, 2], [1, 2], [2, 2], [2, 3], [2, 4], [3, 4], [4, 4]]
Robot 3 path: [[0, 0], [0, 1], [1, 1], [2, 1], [3, 1], [3, 2], [4, 2], [4, 3], [4, 4]]
Robot 4 path: [[0, 0], [0, 1], [1, 1], [2, 1], [3, 1], [3, 2], [4, 2], [4, 3], [4, 4]]
Robot 5 path: [[0, 0], [0, 1], [1, 1], [1, 2], [1, 3], [2, 3], [3, 3], [4, 3], [4, 4]]
Robot 6 path: [[0, 0], [0, 1], [1, 1], [1, 2], [1, 3], [2, 3], [3, 3], [4, 3], [4, 4]]
Robot 7 path: [[0, 0], [0, 1], [0, 2], [1, 2], [2, 2], [2, 3], [2, 4], [3, 4], [4, 4]]
Robot 8 path: [[0, 0], [0, 1], [0, 2], [1, 2], [2, 2], [3, 2], [4, 2], [4, 3], [4, 4]]
Robot 9 path: [[0, 0], [0, 1], [0, 2], [1, 2], [2, 2], [3, 2], [4, 2], [4, 3], [4, 4]]
```
可以看到,第8个机器人找到了一条从起点到终点的路径。
相关推荐
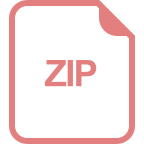
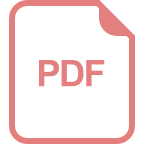














