arduino将字符串数组变成整数数组
时间: 2023-06-01 09:04:36 浏览: 89
您可以使用`atoi()`函数将字符串数组转换为整数数组。下面是一个示例代码:
```c
char strArray[] = "1,2,3,4,5"; // 字符串数组
int intArray[5]; // 整数数组
char *token = strtok(strArray, ","); // 以逗号为分隔符分割字符串
int i = 0;
while (token != NULL) {
intArray[i++] = atoi(token); // 将分割后的字符串转换为整数
token = strtok(NULL, ",");
}
```
在上面的示例中,我们首先使用`strtok()`函数将字符串数组按逗号分隔成多个子字符串。然后,我们使用`atoi()`函数将每个子字符串转换为整数,并将它们存储在整数数组中。
相关问题
arduino 去掉字符串数组中的字符
要从字符串数组中去掉特定的字符,可以使用C++中的`std::remove`函数。这个函数将要删除的字符移动到数组的末尾,并返回一个指向新的末尾位置的迭代器。然后,可以使用`std::string`的`resize`方法将数组的大小缩小到新的末尾位置。
以下是一个示例代码,从字符串数组中删除所有的字母'a':
```c++
#include <algorithm>
#include <string>
int main() {
char arr[] = "hello world";
std::string str(arr);
auto new_end = std::remove(str.begin(), str.end(), 'a');
str.resize(std::distance(str.begin(), new_end));
// 输出结果
for (char c : str) {
Serial.print(c);
}
}
```
以上代码将输出字符串"hello world"中所有'a'字母被删除后的结果。
arduino查找字符串数组中包尾所在的位置,其中字符串数组包含0x00
可以使用 Arduino 的字符串函数库中的 `strchr()` 函数来查找字符串数组中包尾所在的位置,包括字符串数组包含的 `0x00` 字符。`strchr()` 函数的原型如下:
```
char *strchr(const char *str, int c);
```
其中,`str` 参数是要查找的字符串数组,`c` 参数是要查找的字符。函数返回值是指向第一个匹配到的字符的指针,如果没有匹配到则返回 `NULL`。
以下是一个查找包尾所在位置的例子代码,假设字符串数组为 `buf`,包尾字符为 `0x00`:
```cpp
char *tail = strchr(buf, 0x00);
if (tail != NULL) {
int tailIndex = tail - buf; // 计算包尾所在的位置
// 进行后续处理
}
```
在上面的代码中,`tail` 指向包尾字符的指针,如果找到了包尾字符,则计算出它在字符串数组中的位置,并进行后续处理。
相关推荐
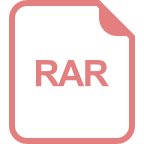
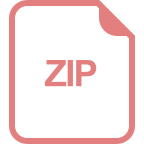












